So, if you’re a Dot NET developer and you are looking to do JumpStart with JAVA then my friend you are just at the right place.
Sometimes you do not live with one language. Especially if you are working for an organization who is working in different dimensions.
Let’s start by viewing a small clip;
So, if we talk about moving to JAVA from .NET then you must remember the concepts of Programming, development techniques which you were using during your Dot NET development. Moreover, as both languages are Object oriented or what we can say OOP based then you must know those concepts including Data Structures and Algorithms.
Perhaps if you’re working upon some specific framework like in UWP or Windows Phone development and you want to move to android then that is the little bit more interesting. For that, you must lead to more hands-on with platform specific stuff.
Although latest platforms start supporting you in a way that you can build cross-platform apps by staying into one language perhaps up till now it’s not much compatible. You’ve to go move beyond that and work upon platform-specific languages.
So, if you’re moving to JAVA from Dot NET then my friend you want to actually play outside Microsoft services.
So, let’s do it!
Again, I’m not talking about which language or platform is good or bad. Just moving to do hands-on with JAVA.
So, to do hands on with JAVA, you simply have to install and configure the JAVA environment on your machine. Simply install JVM, JRE and your favorite IDE and complete the configuration as per required for JAVA.
For the understanding of Java Virtual Machine, Java Runtime Environment and how JAVA code actually works, you can better search for any other places.
For the understanding of different frameworks in JAVA, you can move to the details in other places. Here we’ll be discussing about the Spring MVC framework along with RESTful APIs.
So, let’s get started with Starting line;
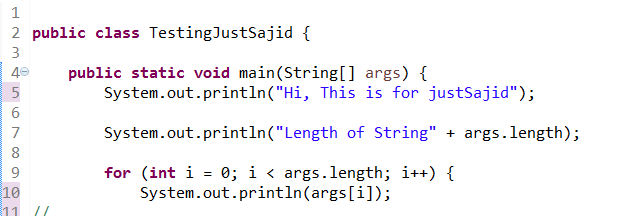
You see, just like C#, we have main method here. We’ve “static” method which means don’t need to make the instance of the class. We’ve “void” mean the method is not returning anything. And we have “String[ ] args” means we can pass arguments, “[ ]” mean arguments are in Array.
Just like C#, everything is an object in JAVA.
Declaration of Variables are same;

Same primitives, complex Variables.
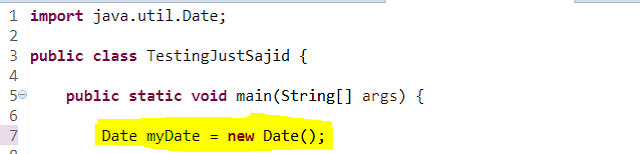
And if we’re talking about primitive data types then it’s the same here;
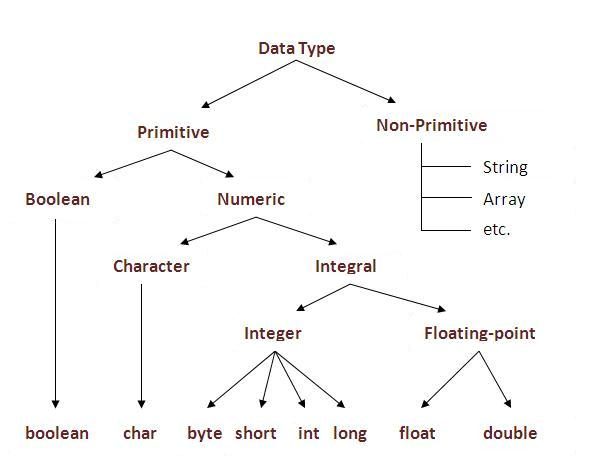
And their values are also same;
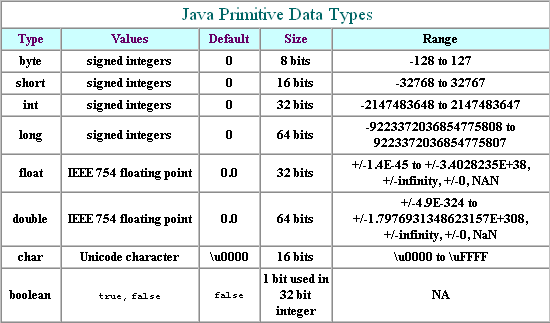
Similarly, we’ve operators in JAVA;
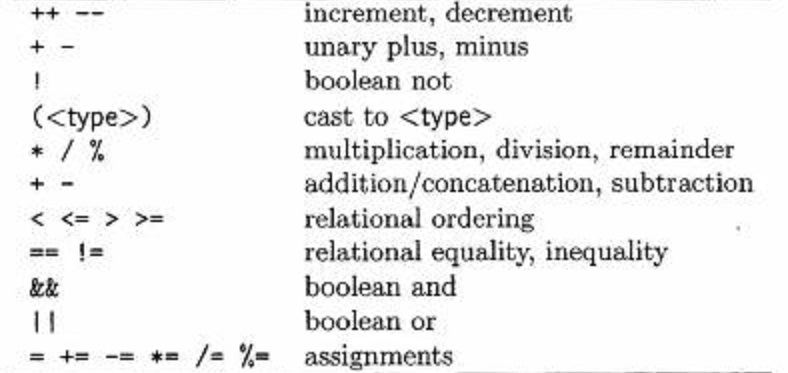
And if we talk about conditional and iteration code then it’s also the same in JAVA.
We’ve if, else, Switch-Case, for, while, do-while same like in C#.
If we talk about the concept of Method Overloading, it’s also the same. We can easily create methods with the same name but having different Signatures.
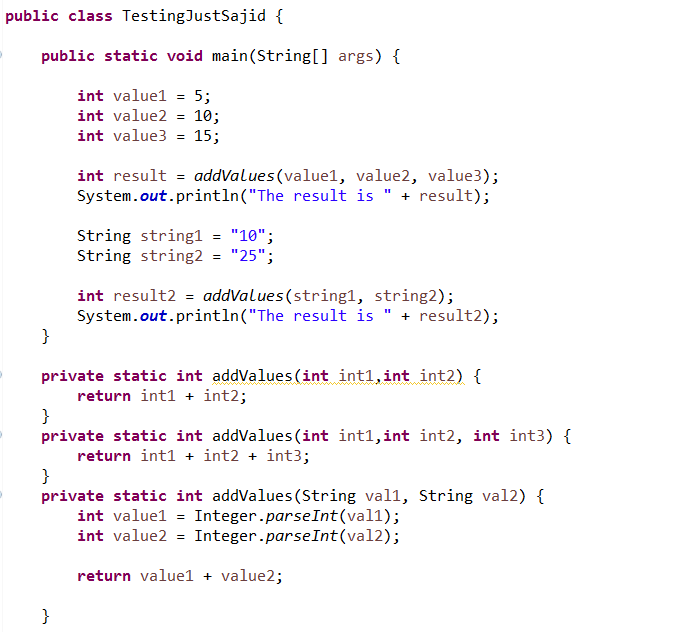
Hence you will be able to overload the method as per circumstances.
If we talk about Passing arguments then just like C#, in JAVA we always pass arguments by value, i.e. we’re always creating a new instance of the value instead of function (Except in certain conditions).
Whenever we pass arguments by tvalue that means we are passing a variable in as an argument to the function. And within the function, there is a new copy of the value.
i.e. The value outside the method and value inside the method are separated and due to which any changes we make inside the function won’t be reflected in the original value;
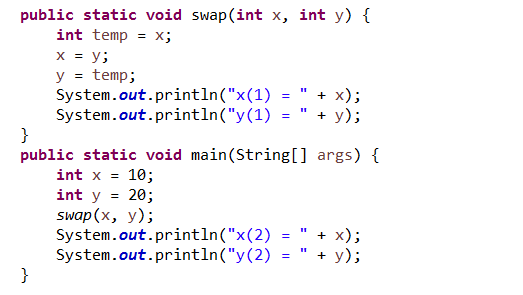
Expected output would be;
x(1) = 20
y(1) = 10
x(2) = 10
y(2) = 20
So logically when we pass the arguments by reference then variable outside the function and the variable inside the function are the same variable and due to which any changes we make inside the function would reflect in the original value, one the function is completed.
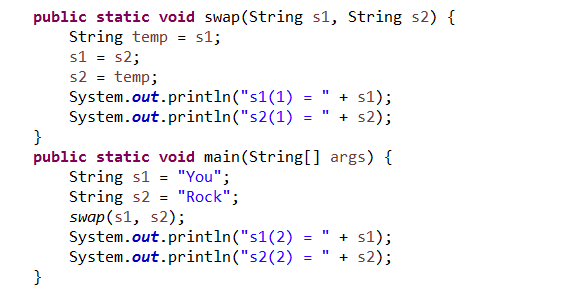
s1(1) = Rock
s2(1) = You
s1(2) = You
s2(2) = Rock
If we talk about exception handling and Debugging, then everything is same in JAVA. Same type of errors, same Try-Catch and Finally blocks;
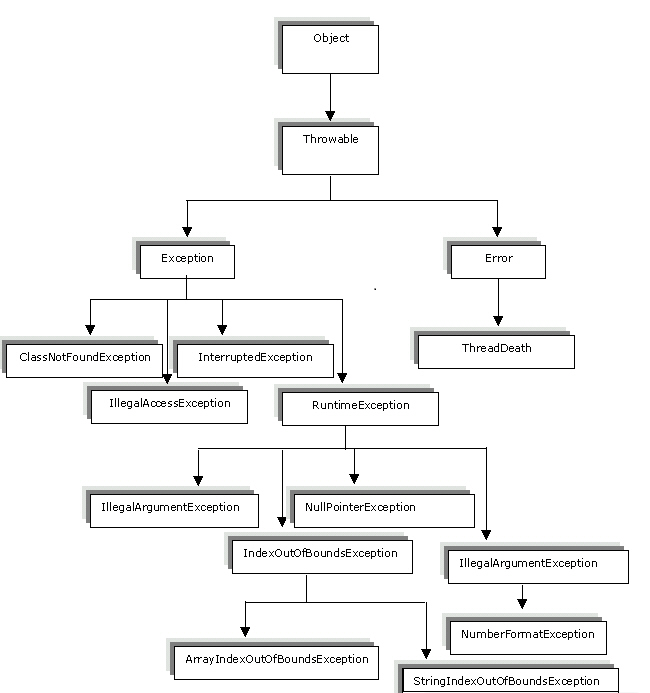
Now, if we talk about the Object-Oriented Programming then all concepts are same.
Encapsulation mean that we are packaging complex functionality to make it easy to use. As being a good programmer we have to break our code into pieces into individual classes to get big advantages. Like we can restrict access to different parts or functions of a class. And we don’t have to tell user about the date, i.e. who is calling a particular class and whether the data is being stored as an array or a collection of some kind or any other object.
We simply provide the method that the user of that class can call and all the complexity is hidden.
So the main benefits of encapsulation are following;
- Breaking functionality into small maintainable units | Easy to find bugs.
- Grouping functions & Data Together.
- Supporting Testing of Software at a granular level.
Now if we talk about class instance and instance variables then the same concept is here. We create instance or object of a class to call the method of that class.
And we use instance variables are used by class instances or objects to store their states. Variables which are defined without the STATIC keyword and are Outside any method declaration are Object specific and are known as instance variables. They are called instance variables because their values are the instance (object) specific and are not shared among instances.
The concept of Constructors is same in JAVA. Used when we create an instance of a class. When we refer to any variable field, prefix it with word “this”. “this” in java means the current instance of a current class. (e.g. this.variable)
In case of more than version of its arguments, we distinguish them by the number or DataType of Arguments.
The concept of Getter and Setter methods are same in JAVA. As in OO development, fields should be private and data should only be accessed through what we call Getter and Setter Method.
In C#, we can use the words set and get before method properties.
In JAVA, we can use explicit setter and getters that are methods that start with word “get” as part of the method name.
For Getter Method; It always has a return data type, which always matches the original data type;
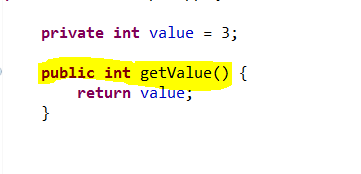
For Setter Method; it has a void return type and has a prefix of set before the field name. Perhaps in arguments, its receives a value that matches the data type of field and then it sets the field using “this” to distinguish the field from arguments.
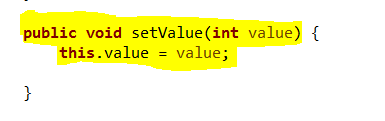
Further, we can set the fields of class through these methods. When we wrap the access of Data in a setter, it lets you change the rules very easily.
In JAVA, we do not have support of explicit ‘cost’ or ‘constant’ keyword, so instead we typically set constant values using a combination of “static” and “final” keyword, which mean the value can’t be changes once it’s been set (and in case of change it cause error).
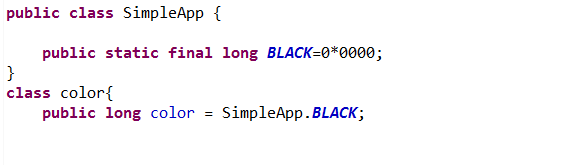
Now the favorite part. The concept of Inheritance and Polymorphism is also similar in JAVA.
Simply used “extend” keyword and easily inherit the parent class. : – )
Like C#, each new class can extend or inherit functionality from only one other class. Perhaps in some cases we might need to do inherit from multiple places. Functionally, there is no multiple inheritance in JAVA, perhaps we can do want to calling interfaces and fulfilled our needs. In this case, we can call maximum one class by using ‘extend’ keyword and along with that, we call one or more interfaces by using “implements” keyword.
Now for the understanding, you can see the details of interfaces, abstract class and when and where we can use them in different scenarios.
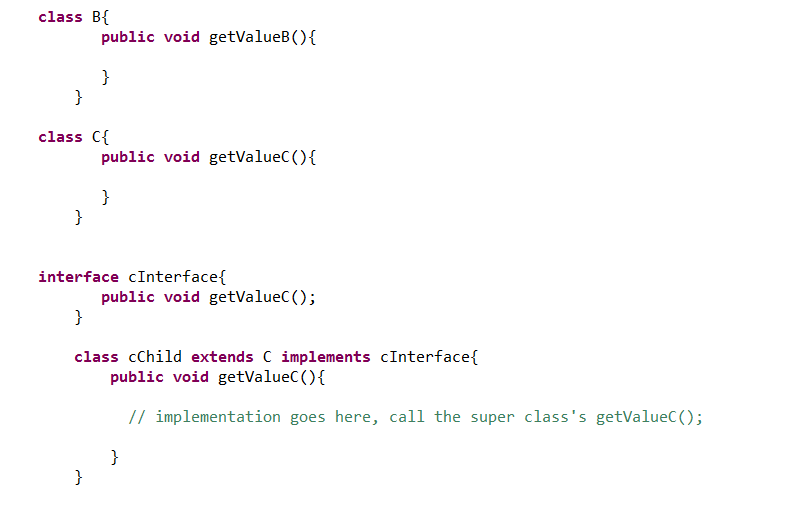
Now, for polymorphism, we need do discuss about overloading and overriding. As discuss earlier about overloading, the overloaded methods may have different return types. The return type alone is insufficient to distinguish two versions of a method.
It allows users to achieve compile-time polymorphism. An overloaded method can throw different exceptions and it can have different access modifiers.
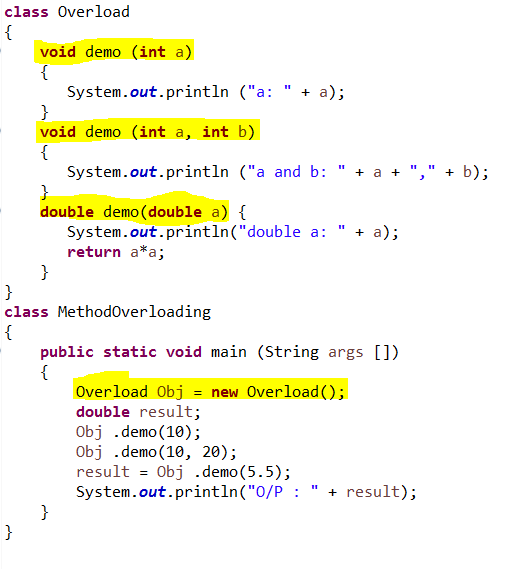
Here the method demo() is overloaded 3 times: first having 1 int parameter, the second one has 2 int parameters and the third one is having double arg. The methods are invoked or called with the same type and the number of parameters used.
Following things should be considered during Method overloading;
- Overloading can take place in the same class or in its sub-class.
- Constructor in Java can be overloaded
- Overloaded methods must have a different argument list.
- The overloaded method
should always be the part of the same class(can also take place in subclass), with the same name but different parameters. - The parameters may differ in their type or number, or in both.
- They may have the same or different return types.
- It is also known as compile time polymorphism.
Now, the Method Overriding is more interesting.
Here, the Child class has the same method as of base class. In such cases, child class overrides the parent class method without even touching the source code of the base class.
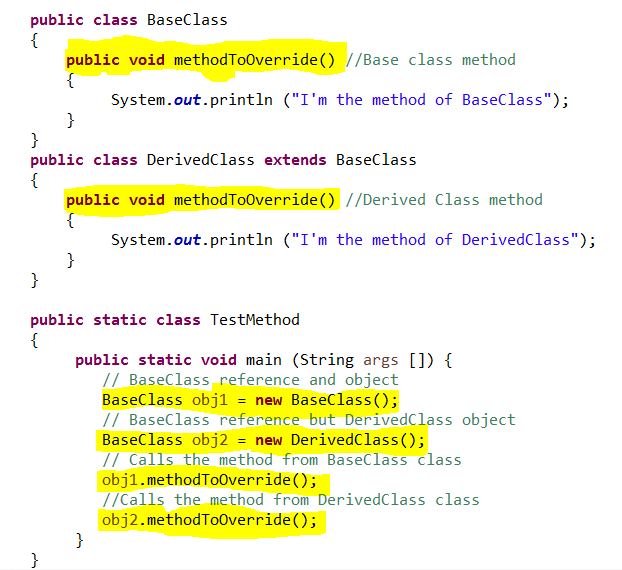
Following things should be considered during Method overloading;
- applies only to inherited methods
- object type (NOT reference variable type) determines which overridden method will be used at runtime
- Overriding method can have the different return type.
- Overriding method must not have more restrictive access modifier
- Abstract methods must be overridden
- Static and final methods cannot be overridden
- Constructors cannot be overridden
- It is also known as Runtime polymorphism.
If we talk about the major differences in the JAVA project structure the one of the big difference is that, The mighty Visual Studio provide us great support to manage the project in almost every way. Perhaps with Eclipse, we have freedom to manage our project in almost every why.
One of the big things is “Package”. Most Classes in a Java App are placed in Packages or subfolder of the project.
As an app gets larger, it’s very important to organized these classes in groups. We call these group as packages but they represent actual physical folder on disk.
In the initial projects, in our src, we have (default packages) where we insert our main class. Perhaps for other classes like helper-classes in app, we have to create new package [helper] and insert our helper class in it.
In large scale, JAVA development environments packages named typically are structured using a prefix of company’s domain in Reverse-Domain-Order. E.g. com.justSajid
The reason to do this so that we can eliminate any possible conflicts with other classes in JAVA environment. And we create universal uniqueness.
JAVA compilation doesn’t create a single monolithic file. Instead, our runtime app consists of a whole bunch of compiled classes and it up to JVM to configure out which classes needed at runtime.
Now, if we talk about the concepts of Data Structures, then all concepts including Stack, Ques, Linked List, Trees and Hashing etc are same. So, you can better consider to review these concepts across the internet.
So, I believe we have enough talk about the comparison of JAVA with C# in all programming concepts. As both as OO languages definitely you see the same flow of programming in both languages.
As per casual discussion with programming experts, it’s very clear that the major difference you can see is that, C# belongs to Microsoft and JAVA is belongs to Oracle (nowadays). So, Microsoft customized C# as per their own ease and compatibility with their tools and platforms. One big reason of C# big hit is Microsoft Visual Studio. I truly believe that, most of the developers start working on C# and .NET only because of Microsoft Visual Studio. As it is world greatest tool or IDE for building any kind of stuff.
As compared to that, JAVA is quite famous among the open source developer community from day one. It gives you free hand to dev freely by customizing your techniques according to your own.
Well, it’s the big debate when we are talking about the comparison of C#/.NET with JAVA and never ends. So let’s move forward.
Frameworks;
There are different frameworks available in JAVA. Each framework has its own pros and cons. Developers can use any framework as per then need, requirement and ease.
We’ll be talking about MVC and String along with RESTful services here;