Motivation;
Well, if you want to play with data and deal with complex analytics problems then Python is the best for you. We can use Python for developing complex scientific and numeric apps. Python is designed with features to facilitate data analysis and visualization.
The syntax in Python helps the programmers to do coding in fewer steps as compared to Java or C++. The language founded in the year 1991.
Why Python;
Python is the MOST popular, high-level, open-source programming language! It is a powerful, fast and dynamic programming language that runs everywhere. It’s also interactive, object-oriented, and very easy to learn.
Anyone can create programs with it. It’s particularly great for web development and scientific computing. With its vast libraries, it’s also useful for data visualization and data analytics.
The cool thing about Python is that it has versatile features and fewer programming codes. Further, it provides the language with a higher plethora over other programming languages used in the industry. Some of its advantages are;
- Extensive Support Libraries;
It provides large standard libraries that include the areas like string operations, Internet, web service tools, operating system interfaces, and protocols. Most of the highly used programming tasks are already scripted into it that limits the length of the codes to be written in Python.
- Integration Feature;
Python integrates the Enterprise Application Integration that makes it easy to develop Web services by invoking COM or COBRA components. It has powerful control capabilities as it calls directly through C, C++ or Java via Jython. Python also processes XML and other markup languages as it can run on all modern operating systems through same byte code.
- Improved Programmer’s Productivity
The language has extensive support libraries and clean object-oriented designs that increase two to ten fold of programmer’s productivity while using the languages like Java, VB, Perl, C, C++, and C#.
- Productivity;
With its strong process integration features, unit testing framework and enhanced control capabilities contribute towards the increased speed for most applications and productivity of applications. It is a great option for building scalable multi-protocol network applications.
There’s a lot to go over but by practicing all these concepts you’ll be well on your way to using Python in your own applications. One can easily be up and running with Python in no time! So, let’s get started!
BTW, Python executes with the help of an interpreter instead of the compiler, which causes it to slow down because compilation and execution help it to work normally. On the other hand, it can be seen that it is fast for many web applications too.
Let’s say hay to Python on Visual Studio;
Create Python Project in Visual Studio;
On Visual Studio, simply create a new python Project;
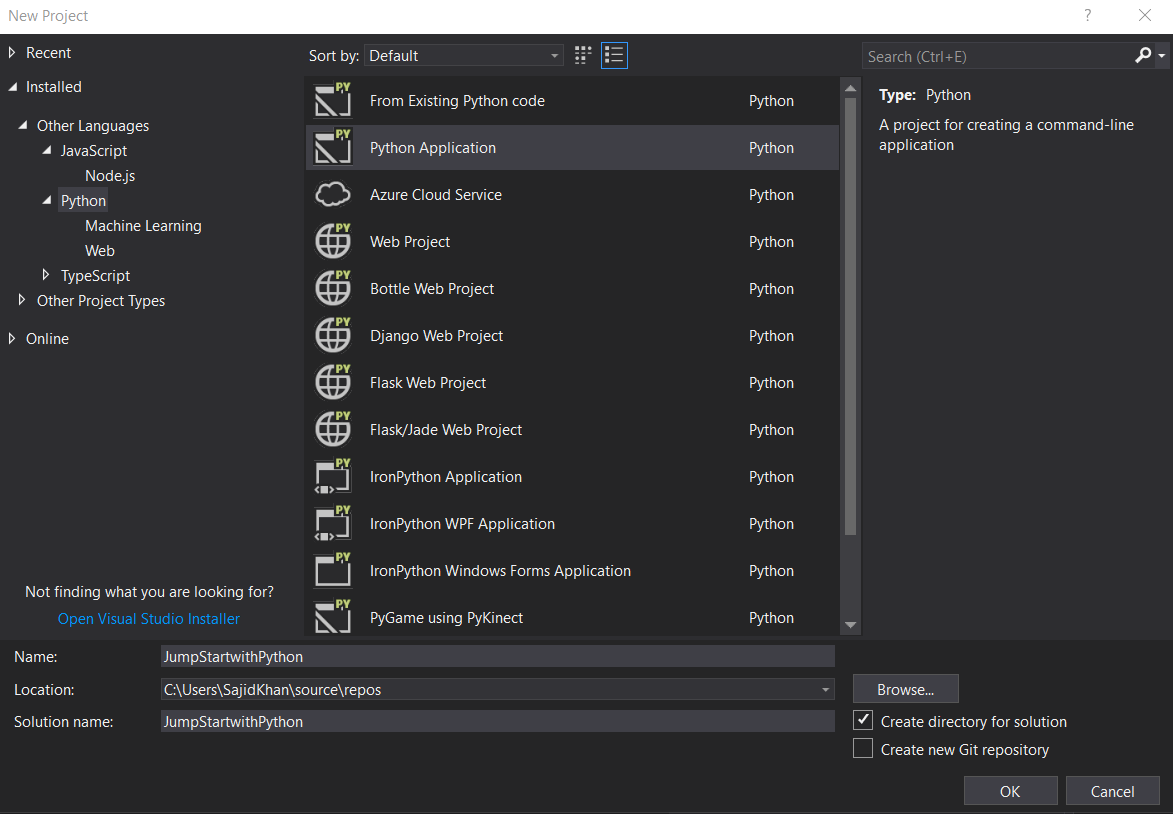
Visual Studio will create a new project template with a proper structure from where we can simply focusing on the python code. Here we would have file hierarchy in the solution explorer including search paths, python environments and all the files that we would be using;
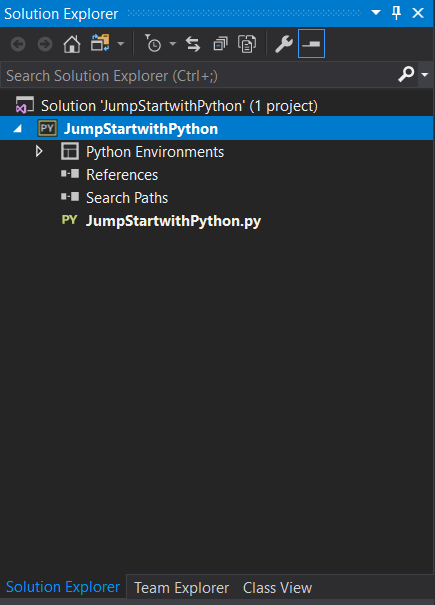
To add new items, we simply add it from the solution explorer;
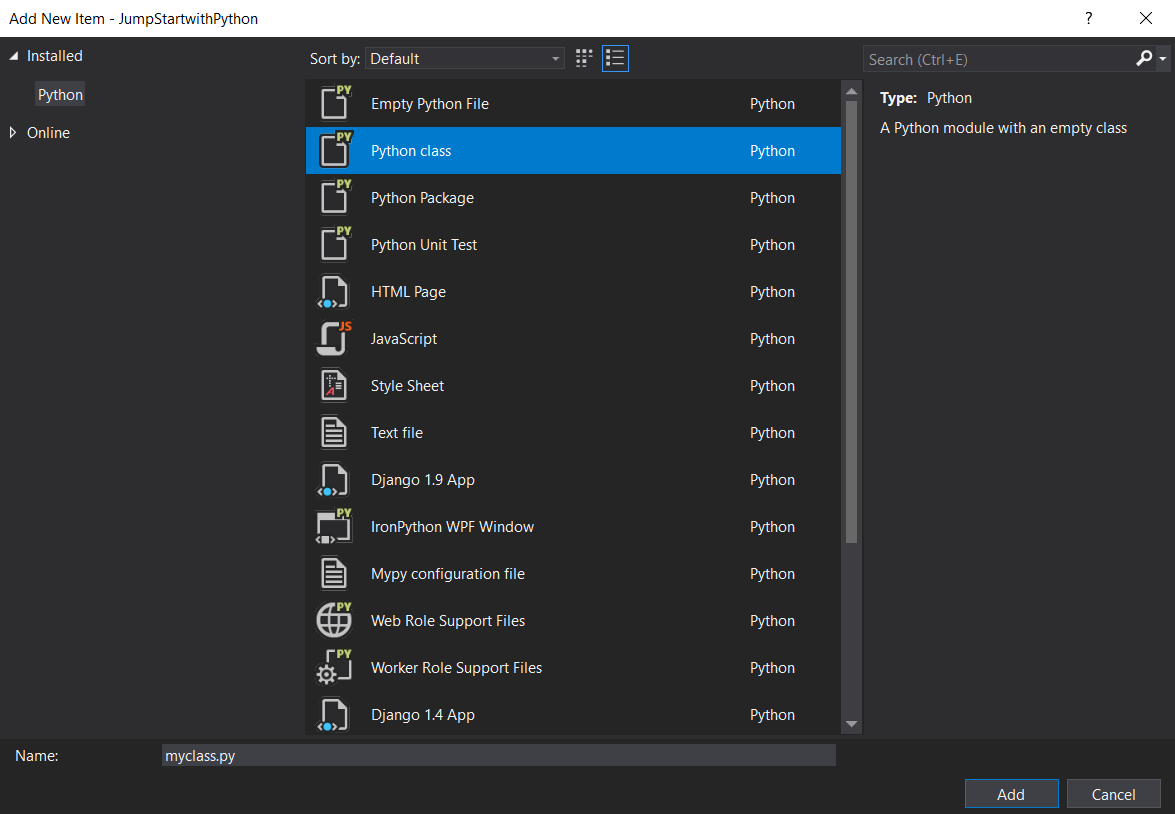
A statement or expression is an instruction the computer will run or execute. Perhaps the simplest program you can write is a print statement. When you run the print statement, Python will simply display the value in the parentheses. The value in the parentheses is called the argument.
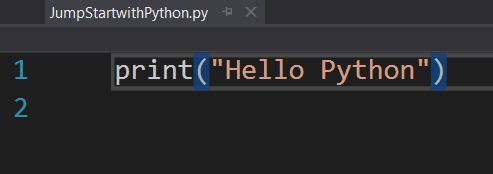
Now if you simply run the program, The statement will execute. The result will be displayed in a console window;
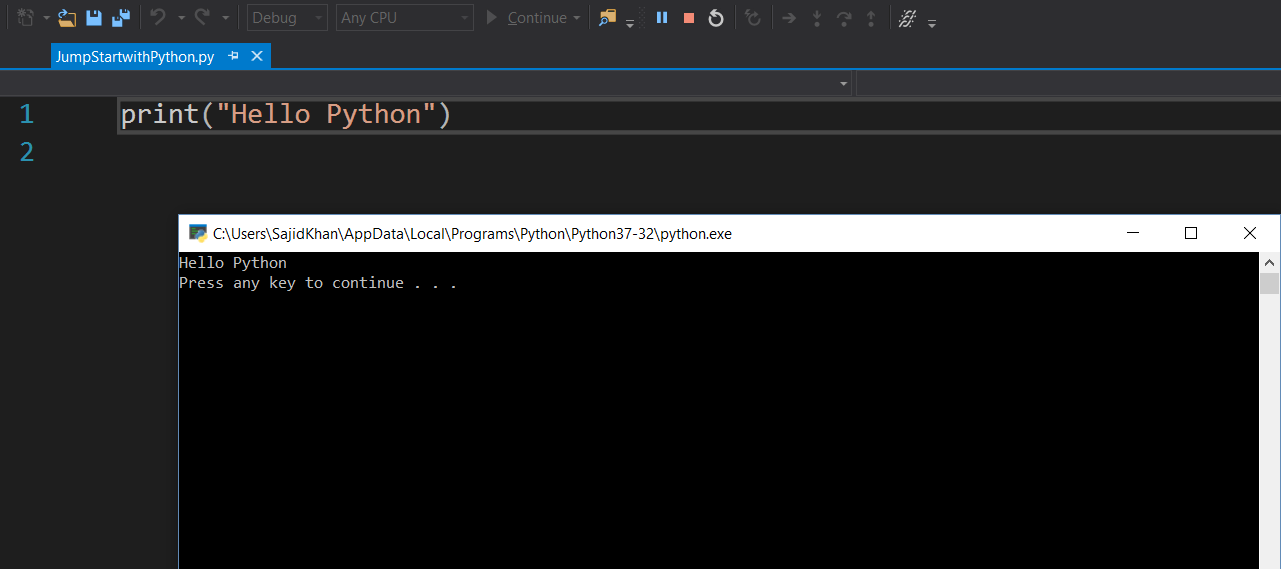
Comments;
It’s customary to comment your code. This tells other people what your code does. You simply put a hash symbol proceeding your comment. When you run the code, Python will ignore the comment.
Errors
A Syntactic error is when Python does not understand your code. For example, if you spell print “frint”, you will get an error
message.
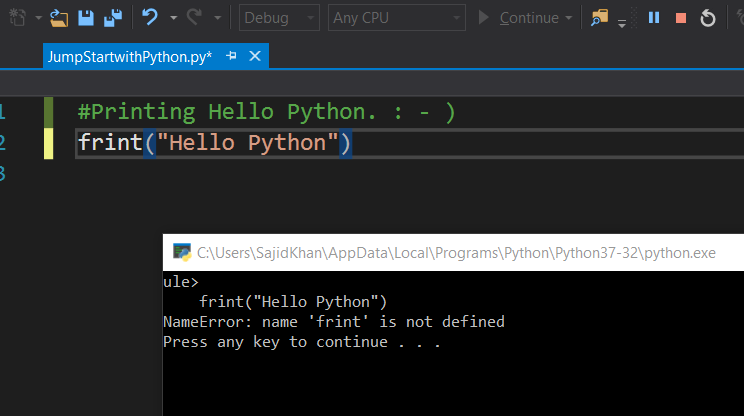
A Semantic error is when your logic is wrong. For example, if you enter Heelo Python instead of Hello Python, you don’t get an error message, but your code is wrong as per expected logic.
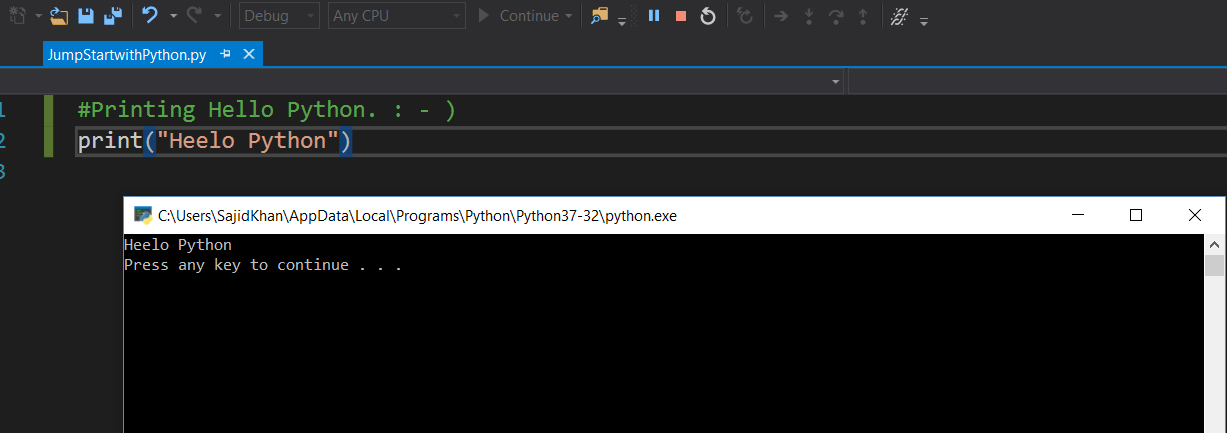
Data Types;
A type is how Python represents different types of data. We can have different types in Python. They can be integers like 11, real numbers like 21.213. They can even be words. Integers, real numbers, and words can be expressed as different data types.
The following chart summarizes three data types for the given examples.
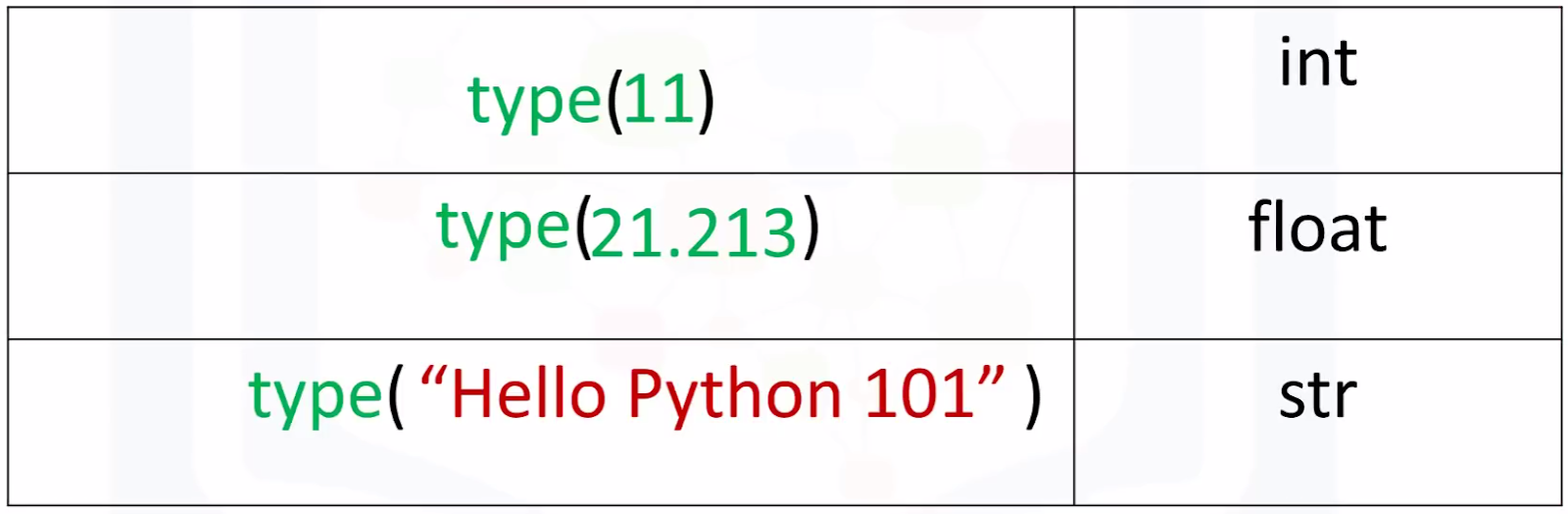
The first column indicates the expression. The second column indicates the data type. We can see the actual data type in Python by using the type command. We can have int, which stands for an integer, and float that stands for float, essentially a real number. The type string is a sequence of characters.
- The integers can be negative or positive. E.g. N = {0, +-1, +-2, +-3, … , +-n}
- Integers can be negative or positive. It should be noted that there is a finite range of integers, but it is quite large.
- The Floats are real numbers. E.g. R = {0, +-0.1, +-0.21, +-0.321, … , +-n }
- They include the integers but also numbers in between the integers. Consider the numbers between 0 and 1. We can select numbers in between them; these numbers are floats.
- Similarly, consider the numbers between 0.5 and 0.6. We can select numbers in-between them; these are floats as well.
- We can continue the process, zooming in for different numbers. Of course, there is a limit,
but it is quite small.
- Type Casting; We can change the type of the expression in Python; this is called type-casting. We can convert an int to a float.
- E.g. we can convert or cast the integer 2 to a float 2. Nothing really changes.
- If we cast a float to an integer, we must be careful. e.g. if you cast the float 1.1 to 1, you will lose some information.
- If a string contains an integer value, we can convert it to int.
- If we convert a string that contains a non-integer value, we get an error.
* You can further do the practice over the Visual Studio, You can convert an int to a string or a float to a string. Boolean is another important type in Python. A Boolean can take on two values. The first value is true, just remember we use an uppercase T. Boolean values can also be false, with an uppercase F. Using the type command on a Boolean value, we obtain the term bool, this is short for Boolean. If we cast a Boolean true to an integer or float, we will get a 1. If we cast a Boolean false to an integer or float, we get a zero. If you cast a 1 to a Boolean, you get a true. Similarly, if you cast a 0 to a Boolean, you get a false.
Mathematical Expression;
Expressions are operations that Python performs. For example, basic arithmetic operations like adding multiple numbers. E.g. 40+50+90. We call the numbers “40” operands and the maths symbols “+” are called operators.
We can perform multiplication operations using the asterisk *. We can also perform division with the forward slash /. E.g. 25/6 is approximately 4.167.
We can also use the double slash for integer division, where the result is rounded. E.g. 25//6 is 4. Be aware, in some cases, the results are not the same as in the regular division.
Python follows mathematical conventions when performing mathematical expressions.
Variables;
We can use variables to store values. E.g. my_variable=1
In this case, we assign a value of 1 to the variable my_variable using the assignment operator. i.e. the equals sign.
We can then use the value somewhere else in the code by typing the exact name of the variable. my_variable:1, we will use a colon do denote the value of the variable.
We can store the results of expressions, for example, we add several values and assign the result to x. x now stores the result. We can also perform operations on x and save the result to a new variable y. We can also perform operations on x and assign the value x.
Strings
In Python, a string is a sequence of characters.
- A string is contained within two quotes. E.g. “justSajid”
- You could also use single quotes. E.g. ‘justSajid’
- A string can be spaces or digits. E.g. ‘just Sajid 007’
- A string can also be special characters. E.g. “£R$£just £!$% Sajid )(*&^”
- We can bind or assign a string to another variable. E.g. Name = “Sajid Ali Khan”
- * It is helpful to think of a string as an ordered sequence. Each element in the sequence can be accessed using an index represented by the array of numbers.
- The first index can be accessed as follows. E.g. Name[0]:M
- We can access index 6. E.g. Name[6]:A
- We can also use negative indexing with strings.
- The last element is given by the index -1. E.g. Name[-1]:n
- The first element can be obtained by index -14. E.g Name[-14]:S – and so on.
- We can bind a string to another variable. Name = “Sajid Ali Khan”, It is helpful to think of a string as a list or tuple.
- Slicing: We can treat the string as a sequence and perform sequence operations.
- E.g. Name[0:4] = Saji or Name[10:12] = Kha
- Stride: We can also input a stride value as follows. Name[::2]:”Sjdaika”
- The 2 indicates we select every second variable.
- SWe can also incorporate slicing. Name[0:5:2]:”Sjd”
- In this case. we return every second value up to index four.
- Slicing: We can use the “len” command to obtain the length of the string. E.g. len(“Sajid Ali Khan”)
- As there are 14 elements, the result is 14.
- Slicing: We can treat the string as a sequence and perform sequence operations.
- We can concatenate or combine strings. We use the addition symbols. The result is a new string that is a combination of both.
- E.g.
- Name = “Sajid Ali Khan”
- Statement = Name + ” is the best”
- The result will be as following; “Sajid Ali Khan is the best”.
- E.g.
- We can replicate the values of a string. We simply multiply the string by the number of times we would like to replicate it;
- E.g.
- 3 * “justSajid “
- The result will be a new strong “justSajid justSajid justSajid”.
- The result is a new string. The new string consists of three copies of the original string.
- This means you cannot change the value of the string.
- E.g. Name = “Sajid Ali Khan”
- Name [0] = “J” –> This is not possible.
- But you can create a new string. For example, you can create a new string by setting it to the original variable and concatenated with a new string.
- E.g.
- Name = Name + ” is the best”.
- Name: “Sajid Ali Khan is the best”.
- The result is a new string that changes from Sajid Ali Khan to “Sajid Ali Khan is the best.”
- E.g.
- E.g. Name = “Sajid Ali Khan”
- E.g.
- Escape Sequences: Strings are immutable. “\” Backslashes represent the beginning of escape sequences.
- Escape sequences represent strings that may be difficult to input.
- For example, backslashes n “\n” represents a new line.
- Similarly, \t represents a tab.
- If we want to place a backslash in your string, use a double backslash “\\”. The result is a backslash “\” after the escape
sequence.
- Escape sequences represent strings that may be difficult to input.
- String Methods:
Strings are sequences and, as such, have apply methods that work on lists and tuples. Strings also have a second set of methods
that just work on strings.
- When we apply a method to the string “A”, we get a new string “B” that is different from “A”.
- E.g.
- A = “This is all about justSajid”
- B = A.upper()
- B:”THIS IS ALL ABOUT JUSTSAJID”
- E.g.
- The method replaces a segment of the string, i.e., a substring with a new string.
- E.g.
- A = “Sajid is the best”
- B = A.replace(‘Sajid’, ‘justSajid’)
- We input the part of the string we would like to change. The second argument is what we would like to exchange the segment with.
- The result is a new string with the segment changed.
- B:’justSajid is the best’
- E.g.
- The method “find” finds the sub-strings.
- The argument is the sub-string you would like to find.
- E.g.
- Name = “Sajid Ali Khan”
- Name.find(‘id’):3
- The output is the first index of the sequence.
- We can find the sub-string Ali.
- E.g. Name.find(‘Ali’):6
- If the substring is not in the string, the output is a negative one.
- E.g. Name.find(‘&*S’):-1
Lists and Tuples:
These are called compound data types and are one of the key types of data structures in Python.
Tuples:
Tuples are an ordered sequence. They are expressed as comma-separated elements within parentheses.
- Here is a Tuple “Ratings”
- Rating = (10, 9, 6, 5, 10, 8, 9, 6, 2)
- These are values inside the parentheses.
In Python, there are different types:
- Strings, e.g. ‘justSajid’.
- Integer, e.g. ’10’.
- float, e.g. ‘1.2’.
They can all be contained in a tuple, e.g. tuple1 = (‘justSajid’, 10, 1.2). But the type of the variable is a tuple, e.g. type (tuple1) = tuple
- Each element of a tuple can be accessed via an index.
- E.g.
- Tuple1[0]:”justSajid”
- The first element can be accessed by the name of the tuple followed by a square bracket with the index number, in this case, zero.
- We can access the second element as follows.
- Tuple1[1]:10
- Similarly, we can access the last element;
- Tuple1[2]:1.2
- In Python, we can use the negative index. E.g Tuple1[-3], Tuple1[-2], Tuple1[3].
- E.g.
- Concatenate Tuples: We can concatenate or combine tuples by adding them. The result is the following with the following index.
- tuple1 = (‘justSajid’, 10, 1.2)
- tuple2 = tuple1 + (‘rock’, 10)
- (“justSajid”, 10, 1.2, “rock”, 10).
- Index [0], [1], [2], [3], [4]
- Slicing: If we would like multiple elements from a tuple, we could also slice tuples.
- E.g.
- If we want the first three elements, we use the following command;
- tuple2[0:3] : (‘justSajid’, 10, 1.2)
- * The last index is 1 larger than the index you want. Like here we’ve got from 0 till the index 2.
- If we want the first three elements, we use the following command;
- E.g.
- We can use the “len” command to obtain the length of a tuple.
- E.g.
- len((“justSajid”, 10, 1.2, “rock”, 10))
- As there are 5 elements, the result is 5.
- E.g.
- Immutable: Tuples are immutable, which means we can’t change them.
- E.g.
- Ratings = (10, 9, 6, 5, 10, 8, 9, 6, 2)
- To see why this is important, let’s see what happens when we set the variable Ratings 1 to ratings.
- Ratings1 = Ratings
- Each variable does not contain a tuple, but references the same immutable tuple object.
- Let’s say we want to change the element at index 2. Because tuples are immutable, we can’t.
- i.e Ratings[2] = 4 (Not possible).
- Therefore, Ratings1 will not be affected by a change in Ratings because the tuple is immutable i.e., we can’t change it.
- We can assign a different tuple to the Ratings variable.
- E.g. Ratings = (2, 10, 1)
- The variable Ratings now references another tuple.
- As a consequence of immutability, if we would like to manipulate a tuple, we must create a new tuple instead.
- For example, if we would like to sort a tuple, we use the function sorted.
- E.g. RatingSorted = sorted(Ratings)
- The input is the original tuple. The output is a new sorted tuple.
- Nesting: A tuple can contain other tuples as well as other complex data types; this is called nesting. We can access these elements using the standard indexing methods.
- If we select an index with a tuple, the same index convention applies.
- E.g. NT = (1,2, (“alpha”, “beta”), (3, 4), (“gemma”, (1, 2)))
- NT[2]:(“alpha”, “beta”)[1] = “rock”
- NT[2][1]=”beta”
- We can apply this indexing directly to the tuple variable NT. It is helpful to visualize this as a tree. We can visualize this nesting as a tree.
- E.g. NT[2][1]=”beta”
- We can even access deeper levels of the tree by adding another square bracket.
- E.g. NT[2][1][0]=”a”
- E.g.
Lists;
Lists are also a popular data structure in Python. Lists are also an ordered sequence.
- E.g. L = [“justSajid”, 10.1, 1991]
- A list is represented with square brackets.
- In many respects lists are like tuples, one key difference is they are mutable (But tuples are Immutable).
- Lists can contain strings, floats, integers.
- We can also nest other lists.
- E.g. L = [“justSajid”, 10.1, [1,2]]
- We also nest tuples and other data structures;
- E.g. L = [“justSajid”, 10.1, (‘A’, 1)]
- The same indexing conventions apply for nesting. Like tuples, each element of a list can be accessed via an index.
- Similarly, we can apply slicing to the lists.
- E.g. L = [“justSajid”, 10.1, 1991, “JS”, 1]
- L[3:5]:[“JS”, 1]
- E.g. L = [“justSajid”, 10.1, 1991, “JS”, 1]
- Concatinate; L = [“justSajid”, 10.1, 1991]
- E.g. L1 = L + [“alpha”, 10]
- L1 = [“justSajid”, 10.1, 1991, “alpha”, 10]
- As Lists are mutable, therefore, we can change them.
- E.g. L.extend([“beta, 10”])
- L = [“justSajid”, 10.1, 1991, “beta”, 10]
- E.g. L = L.append([“alpha”, 10])
- L = [“justSajid”, 10.1, 1991, [“alpha”, 10]]
- E.g. L[0] = “alpha”
- L = [“alpha”, 10.1, 1991]
- E.g. L.extend([“beta, 10”])
- Every time we apply a method, the lists changes. We can delete an element of a list using the “del” command; we simply indicate the list item we would like to remove as an argument.
- E.g. del(L[0])
- L = [10.1, 1991]
- E.g. del(L[0])
- We can convert a string to a list using split.
- E.g. “just Sajid”.split()
- [“just”, “Sajid”]
- E.g. “just Sajid”.split()
- We can also use the split function to separate strings on a specific character, known as a delimiter.
- E.g. “A, B, C, D”.split(“,”)
- [“A”, “B”, “C”, “D”]
- E.g. “A, B, C, D”.split(“,”)
- Aliasing; When we set one variable, B equal to A, both A and B are referencing the same list. Multiple names referring to the same object is known as aliasing.
- Now here is an interesting thing will happen.
- E.g.
- A = [“justSajid”, 10, 1.2]
- B = A
- Now, If we change the first element in “A” to “banana” we get a side effect; the value of B will change as a consequence.
- A [0] = “alpha”
- If we check the first element of B after changing list ”A” we get alpha instead of justSajid.
- E.g.
- You can clone list “A” by using the following syntax.
- B = A[:]
- Variable “A” references one list. Variable “B” references a new copy or clone of the original list.
- Now if you change “A”, “B” will NOT change.
- B = A[:]
- Now here is an interesting thing will happen.
Sets;
They are also a type of collection. Sets are a type of collection. This means that like lists and tuples, we can input different python types.
Unlike lists and tuples, they are unordered. This means sets do not record element position.
Sets only have unique elements. This means there is only one of a particular element in a set.
- Defining Set; To define a set, you use curly brackets. We place the elements of a set within the curly brackets. We notice there are duplicate items.
- E.g.
- Set1 = {“justSajid”, “alpha”, “beta”, “delta”, “justSajid”}
- As we can see there are duplicate items in the Set1. Perhaps when the actual set is created, duplicate items will not be present.
- i.e. Set1 = {“justSajid”, “alpha”, “beta”, “delta”}
- E.g.
- We can convert a list to a set by using the function set; this is called type-casting. We simply use the list as the input to the function set. The result will be a list converted to a set.
- E.g.
- List_1 = [“justSajid”, “alpha”, “beta”, “delta”, “justSajid”]
- Set_1 = set(List_1)
- Set_1: {“justSajid”, “alpha”, “beta”, “delta”}
- E.g.
- Set Operations; Used to change the function set.
- We can add an item to a set using the add method.
- E.g. A.add(“Software”)
- A:{“justSajid”, “alpha”, “beta”, “delta”, “Software”}
- * If we add same item twice, nothing will happen as there can be no duplicates in a set.
- We can also remove an item from a set using the remove method.
- E.g. A.remove(“justSajid”)
- A: {“alpha”, “beta”, “delta”}
- We can verify if an element is in the set using the “in” command as follows.
- E.g. “justSajid” in A
- True
- If we look for an item that is not in the set, we will get a False.
- E.g. “justSajid” in A
- There are lots of useful mathematical operations we can do between sets.
- The Intersection of two sets is a new set containing elements which are in both of those sets.
- The two circles that represent the sets combine; the overlap represents the new set.
- We define the intersection in terms of “and.” In Python, we use the ampersand “&” to find the union of two sets.
- E.g.
- set_1 = {“justSajid”, “alpha”, “beta”, “delta”}
- set_2 = {“alpha”, “beta”, “delta”, “Software”}
- set_3 = set_1 & set_2
- set_3:{“alpha”, “beta”}
- That is containing all the elements in both album set 1 and album set 2.
- E.g.
- Similarly, we can find the Union of the set.
- E,g
- set_1.union(set_2)
- {“justSajid”, “alpha”, “beta”, “delta”, Software}
- E,g
- We can check if a set is a subset using the is subset method.
- E.g.
- set_3.issubset(set_1)
- True
- E.g.
- There is a lot more we can play with Sets.
Dictionaries:
- Dictionaries are a type of collection in Python.
- E.g. A list has integer indexes. These are like addresses. A list also has elements.
- A dictionary has keys and values.
- The key is analogous to the index, they are like addresses but they don’t have to be integers. They are usually characters; the values are similar to the elements in a list and contain information.
- To create a dictionary, we use curly brackets. The keys are the first elements; they must be immutable and unique.
- E.g.
- {“key1”: 1, “key2”:2, “key3”:[333], (‘key4’):5}
- Each key is followed by a value separated by a colon. The values can be immutable, mutable and duplicates.
- Each key and value pair is separated by a comma.
- E.g.
- We can also assign the dictionary to a variable.
- The key is used to look up the value.
- We use square brackets; the argument is the key.
- E.g.
- DICT[“key1”]: 1 // This outputs the value.
- E.g.
- Similarly, we can further play with Dictionaries by applying different methods.
Conditions;
Comparison operations compare some value or operand, then, based on some condition they produce a Boolean.
- Let’s say we assign “a” value of “a” to 6.
- E.g. a = 6
- We can use the equality operator denoted with two equal signs to determine if two values are equal,
- E.g. a == 7
- In this case, as six is not equal to 7, the result is False.
- Perhaps if we performed an equality test for the value 6,
- E.g. a == 6
- As the two values would be equal. So, we would get a true.
Similarly, Consider the following equality comparison operator.
- E.g. i>5
- If the value of the left operand, in this case, the variable “i” is greater than the value of the right operand, in this case, 5, the condition becomes true, or else we get a false.
- Branches;
Branching allows us to run different statements for a different input.
It’s helpful to think of an “if statement” as a locked room:
- If the statement is true,
- We can enter the room, and your program can run some predefined task.
- If the statement is false,
- Our program will skip the task.
In Python, we use the following syntax for the conditional statement.
if (age>7):
print(“You can enter”)
print(“move on”)
Similarly for else statement;
if (age>7):
print(“You can enter”)
else:
print(“go back”)
print(“move on”)
Else if syntex is also similar; elseif(“age==8”): print (“please come”)
- Logic Operators; They take Boolean values and produce different Boolean values.
- Its logic is the same as we use in our mathematics.
- I.e. and(), or( ),
- If (Year<1992) or (Year>1990)
Loops;
Before we talk about loops, let’s go over the range function. The range function outputs an ordered sequence as a list “i”. If the input is a positive integer, the output is a sequence; the sequence contains the same number of elements as the input but starts at zero.
- range(N) = [0…, n-1]
- E.g. range(3) = [0, 1, 2], range(10, 15) = [10, 11, 12, 13, 14]
- If the range function has two inputs where the first input is larger than the second input, the output is a sequence that starts at the first input.
Loops perform a task over and over. The syntax in Python is following
- for i in variable:
- x=x+1
- print(“Hello For Loop”)
“While loops” are similar to “for loops”, but instead of executing a statement a set number of times, a while loop will only run if a condition is met.
Let’s say we would like to copy all the orange squares from the list squares to the list new squares, but we would like to stop if we encounter a non-orange square.
E.g.
- sum = 0
- i = o
- while i < 10:
- sum = sum + i
- print sum
- i = i + 1
- for i in range (10):
- sum = sum + i
- print sum
Functions:
Functions take some input then produce some output or change. The function is just a piece of code we can reuse. We can implement our own function, but in many cases, you use other people’s or predefined functions.
In some cases, you just have to know how the function works and in some cases how to import the functions.
def f1 (input):
“””Add 1 to Input”””
output = input + 1;
return output
We can think of the process like this: when we call the function f1, we pass input to the function. These values are passed to all those lines of code you wrote.
This returns a value; you can use the value. For example, you can input this value to a new function f2. When we call this new function f2, the value is passed to another set of lines of code. The function returns a value. The process is repeated passing the values to the function you call.
You can save these functions and reuse them, or use other people’s functions.
We can run the code using some input and get an output. If we define a function to do the task we just have to call the function.
Python has many built-in functions; you don’t have to know how those functions work internally, but simply what task those functions perform.
- The function “len” takes an input of type sequences, such as a string or list, or type collection, such as a dictionary or set, and returns the length of that sequence or collection. Consider the following list.
- rating = [10.0, 8.6, 9.5, 7.0, 7.0, 9.6, 9.0, 9.6]
- L = len(rating)
- The len function takes this list as an argument, and we assign the result to the variable L. The function determines there are 8 items in the list, then returns the length of the list, in this case, 8. The function sum takes in an iterable like a tuple or list and returns the total of all the elements.
- L:8
- Similarly;
- S = sum(rating)
- S:70.3
There are two ways to sort a list. The first is using the function sorted. We can also use the list method sort. Methods are similar to functions.
Let’s use this as an example to illustrate the difference. The function sorted Returns a new sorted list or tuple.
- E,g,
- sorted_rating = sorted(rating)
- sorted_rating: [7.0, 7.0, 8.6, 9.0, 9.6, 9.6, 10.0]
- The result is a new sorted list. If we look at the list rating, nothing has changed. Generally, functions take an input, in this case, a list. They produce a new output, in this instance, a sorted list.
- If we use the method sort, the list album ratings will change and no new list will be created.
- rating.sort()
- When we apply the method sort to the list, the list album rating changes. Unlike the previous case, we see that the
list album rating has changed. In this case, no new list is created.
- When we apply the method sort to the list, the list album rating changes. Unlike the previous case, we see that the
- rating: [10.0, 8.6, 9.5, 7.0, 7.0, 9.6, 9.0, 9.6]
- rating.sort()
- sorted_rating = sorted(rating)
Now let’s play by creating our own functions.
def add1 (a):
b = a+1
return b
- After we define the function, we can call it.
- E.g.
- add1 (5)
- The function will add 1 to 5 and return a 6.
- c = add1 (10)
- C:11
- add1 (5)
- E.g.
- Multiple Parameters; A function can have multiple parameters.
- def Mult (a,b)
- c = a*b
- return c
- Now if we do this; Mult (2, 3) the result would be 6.
- If we pass in the integer two and the string “justSajid;
- Mult (2, “justSajid “)
- The string justSajid is repeated two times; Mult: “justSajid justSajid”
- This is because the multiplication symbol can also mean repeat a sequence.
If you accidentally multiply an integer and a String instead of two integers, you won’t get an error. Instead, you will get a String, and your program will progress, potentially failing later because you have a String where you expected an integer.
In many cases a function does not have a return statement. In these cases, Python will return the special “None” object.
- Practically, if your function has no return statement, you can treat it as if the function returns nothing at all.
- E.g.
- def jS(): ‘justSajid’
- jS()
- ‘justSajid’
- def NoWork(): pass
- print (NoWork())
- None
- * Python doesn’t allow a function to have an empty body, so we can use the keyword pass, which doesn’t do anything, but satisfies the requirement of a non-empty body. If we call the function and print it out, the function returns a None.
- In the background, if the return statement is not called, Python will automatically return a None. It is helpful to view the function NoWork with the following return statement.
- E.g.
Usually, functions perform more than one task.
E.g
- def add2(a):
- b = a + 1
- print (a, “plus one equals “, b)
- return b
add2(2)
- 2 plus one equals 3.
- 3
- Loops in Functions;We can use loops in functions.
- E.g.
- def printStuff(Stuff):
- for i,s in enumerate(Stuff):
- print(“Item”, i , “Rating is “, s)
- rating = [10.0, 8.5, 9.5]
- printStuff(rating)
- The output comes
- Item 0 Rating is 10
- Item 1 Rating is 8.5
- Item 2 Rating is 9.5
- * This function prints out the values and indexes of a loop or tuple. We call the function with the list album ratings as an input. The function will print the first index and the first value in the list. We continue iterating through the loop. The values of i and s are updated. The print statement is reached.
- E.g.
Variadic parameters allow us to input a variable number of elements.
- def AllNames (*names):
- for name in names:
- print (name)
- Consider the following function. The function has an asterisk on the parameter names.
- AllNames (“justSajid”, “AC/DC”, “alpha”)
- When we call the function, three parameters are packed into the tuple names. We then iterate through the loop; the values are printed out accordingly.
- AllNames: [“justSajid”, “AC/DC”, “alpha”]
- If we call the same function with only two parameters as inputs, the variable names only contain two elements. The result is only two values are printed out.
- Variable Scope: The scope of a variable is the part of the program where that variable is accessible. Variables that are defined outside of any function are said to be within the global scope, meaning they can be accessed anywhere after they are defined.
- A variable defined in the global scope is called a global variable. When we call the function, we enter a new scope. We pass as an argument to the function.
- As compare to a global variable, Local variables only exist within the scope of a function.
- If a variable is not defined within a function, Python will check the global scope.
- If we define the variable in the global scope, then call the function, Python will see there is no value for the variable. As a result, python will leave the scope and check if the variable exists in the global scope.
Objects;
Python has many different kinds of data types: Integers, Floats, Strings, Lists, Dictionaries, Booleans. In Python, each is an object.
- Every object has the following:
- A Type
- An internal data representation (a blueprinting)
- A set of procedures for interacting with the object (the method)
- An object is an instance of a particular type.
Every time we create an Integer, we are creating an instance of type integer, or we are creating an integer object. Similarly, every time we create a list, we are creating an instance of type list, or we are creating a list object.
We can find out the type of an object by using the type command;
- type()
Classes
A class or type’s methods are functions that every instance of that class or type provides. It’s how we interact with the object.
- We have been using methods all this time, for example, on lists.
- Sorting is an example of a method that interacts with the data in the object.
- Consider the list Rating.
- E.g.
- Rating = [10, 9, 6, 5, 10, 8, 9, 6, 2]
- The data is a series of numbers contained within the list.
- The method sort will change the data within the object. We call the method by adding a period at the end of the object’s name and the method’s name we would like to call with parenthesis.
- Rating.sort()
- E.g.
- The data contained in the list is a sequence of numbers. When we call the sort method, this changes the data contained in the object. You can say it changes the state of the object.
- Consider the list Rating.
- Sorting is an example of a method that interacts with the data in the object.
We can create our own class;
- The class has data attributes.
- The class has methods.
- We then create instances or instances of that class or objects.
- The class data attributes define the class.
- class Name (object):
- To create the class, we will need to include the class definition.
- i.e the word “class“
- This tells Python you are creating your own class.
- Then the name of the class, i.e. “Name“.
- In parenthesis, you will place the term “object“. This is the parent of the class.
- To create the class, we will need to include the class definition.
- class Name (object):
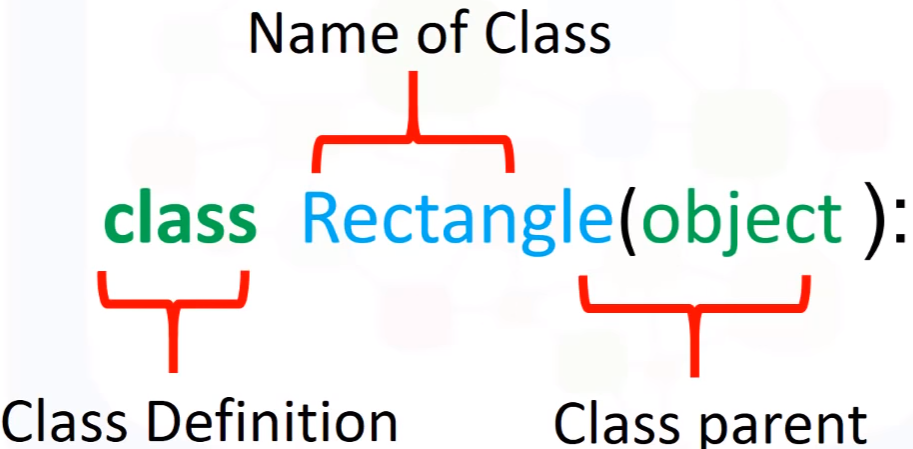
Classes are outlines we have to set the attributes to create objects.
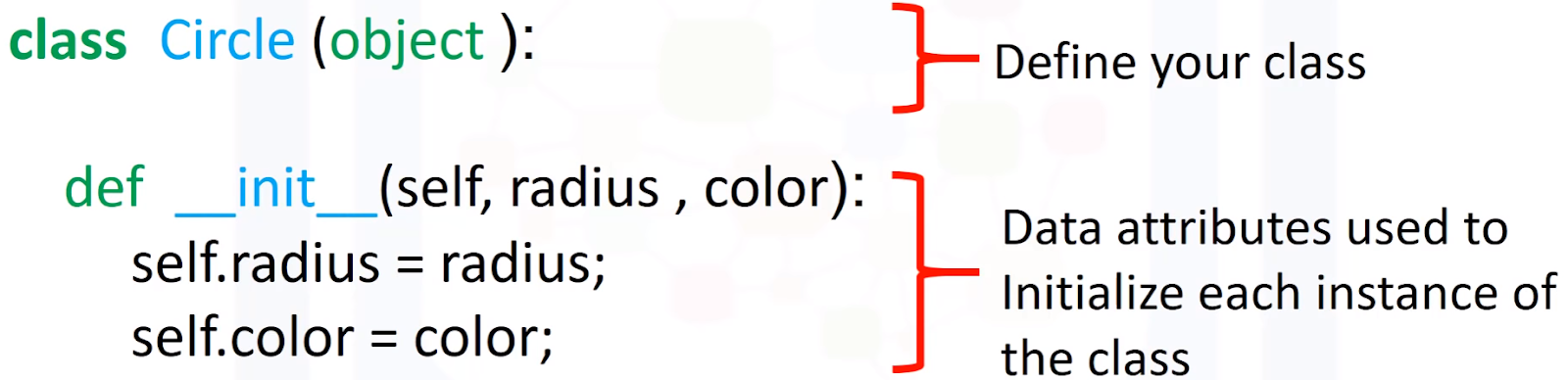
Here,
- The function “_init_” is a constructor. It’s a special function that tells Python you are making a new class.
- The ‘radius’ and ‘color’ parameters are used to initialize the radius and color data attributes of the class instance.
- The ’self’ parameter refers to the newly created instance of the class.
- * It is helpful to think of self as a box that contains all the data attributes of the object.
Now, after we have created the class, in order to create an object of the class circle, we introduce a variable;
- RedCircle = Circle (10, “red”)
- This will be the name of the object. We create the object by using the object constructor. I.e. “RedCircle”
- The object constructor consists of the name of the class, as well as the parameters.
- These are the data attributes.
- When we create a Circle object we call the code like a function. The arguments passed to the Circle constructor are used to initialize the data attributes of the newly created Circle instance.
- C1 = Circle (10, “red”)
- C1.radius
- Typing the object’s name followed by a dot and the data attribute name gives us the data attribute value, for example, radius. In this case, the radius is 10. We can do the same for color.
- C1.radius
In Python, we can also set or change the data attribute directly.
- E.g.
- C1 = Circle (10, “red”)
- C1.color = “blue”
- C1.color
- “blue”
- // Typing the object’s name, followed by a dot and the data attribute name, and set it equal to the corresponding value. We can verify that the color data attribute has changed.
- Methods in Class
Usually, in order to change the data in an object, we define methods in the class.
We have seen how data attributes consist of the data defining the objects. Methods are functions that interact and change the data attributes, changing or using the data attributes of the object.
- E.g. we would like to change the size of a circle; this involves changing the radius attribute.
- def add_radius(self, r)
- self.radius = self.radius + r
- We add a method “add_radius” to the class Circle.
- The method is a function that requires the self as well as other parameters.
- In this case, we are going to add a value to the radius;
- we denote that value as r. We are going to add r to the data attribute radius.
- In this case, we are going to add a value to the radius;
- We can also add default values to the parameters of a class’s constructor.
The “dir” function is useful for obtaining the list of data attributes and methods associated with a class.
- dir (NameOfObject):
- The object you’re interested in is passed as an argument.
- The return value is a list of that object’s data attributes.
- The attributes surrounded by underscores are for Internal Use and you shouldn’t have to worry about them.
- The regular-looking attributes are the ones you should concern yourself with, these are the object’s methods and data attributes.
There is a lot more we can do with objects in Python. Let’s keep playing with further examples for better hands-on. : – )
Conclusion:
So this is just the JumpStart programming with Python. One who reviewed thus with complete focus would have a good understanding of python basic programming on his fingertips.
* The Blog post was compiled with the help of the python course available at Cognitive class. It is highly recommended to review the course from here; https://cognitiveclass.ai/courses/python-for-data-science/