Motivation
In order to do proper hands-on with a new language, it’s important to understand each part of it by applying live examples. Hence its syntax fully clear.
Let’s start by playing with functions;
Functions
- Simply create an add_numbers a function that takes two numbers and adds them together;
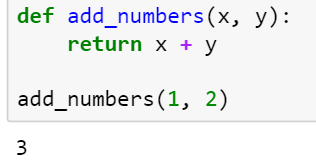
- add_numbers updated to take an optional 3rd parameter.
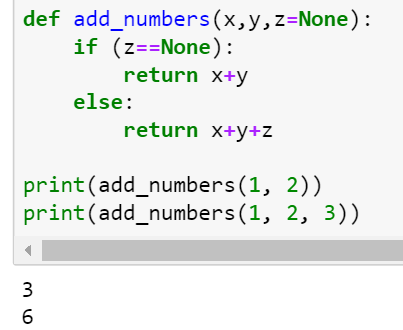
- add_numbers updated to take an optional flag parameter.
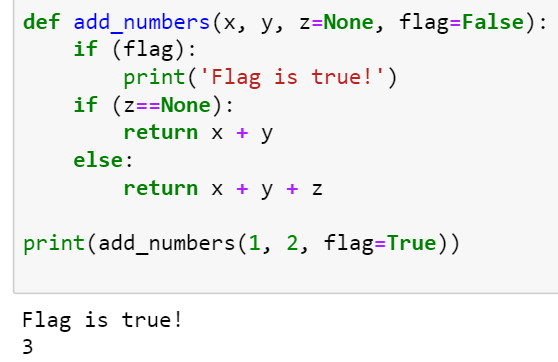
- Assign function add_numbers to variable add.
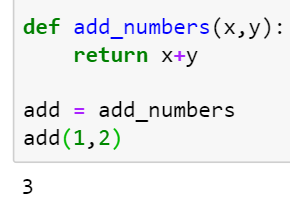
Types and Sequences
- Tuples are an immutable data structure (cannot be altered).
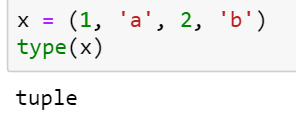
- Lists are a mutable data structure.
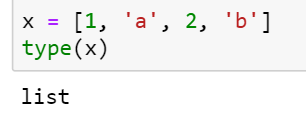
- Use append to append an object to a list.
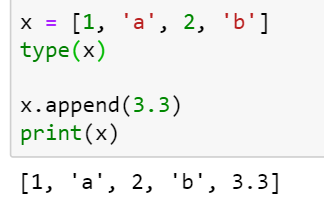
- And by using the indexing operator.
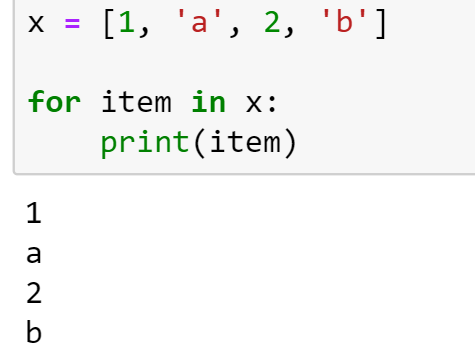
- Use + to concatenate lists
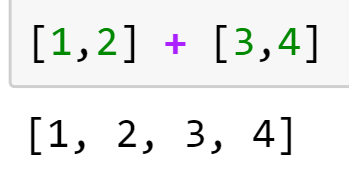
- Use * to repeat lists
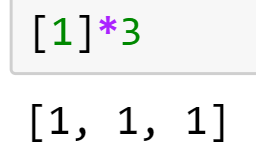
- Use the in operator to check if something is inside a list.
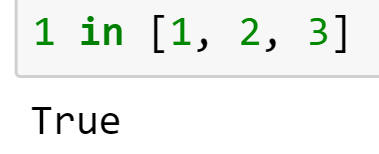
- Let’s look at the strings. Use bracket notation to slice a string.
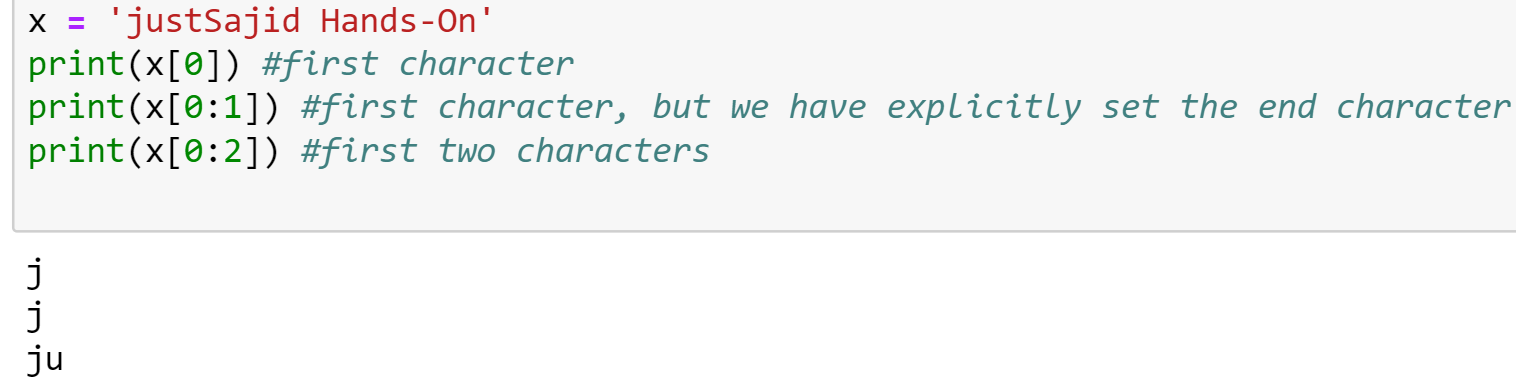
- To return the last element of the string.
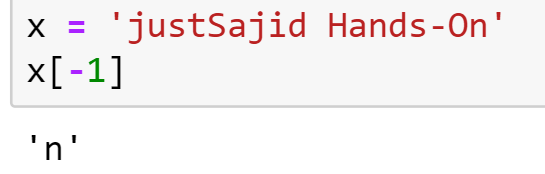
- This will return the slice starting from the 4th element from the end and stopping before the 2nd element from the end.
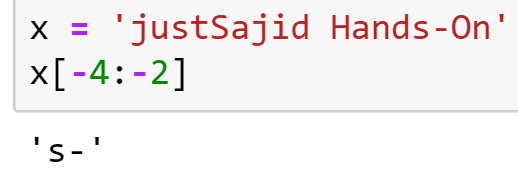
- This is a slice from the beginning of the string and stopping before the 3rd element.
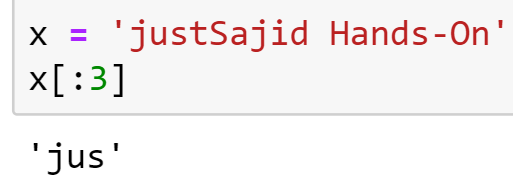
- And this is a slice starting from the 4th element of the string and going all the way to the end.
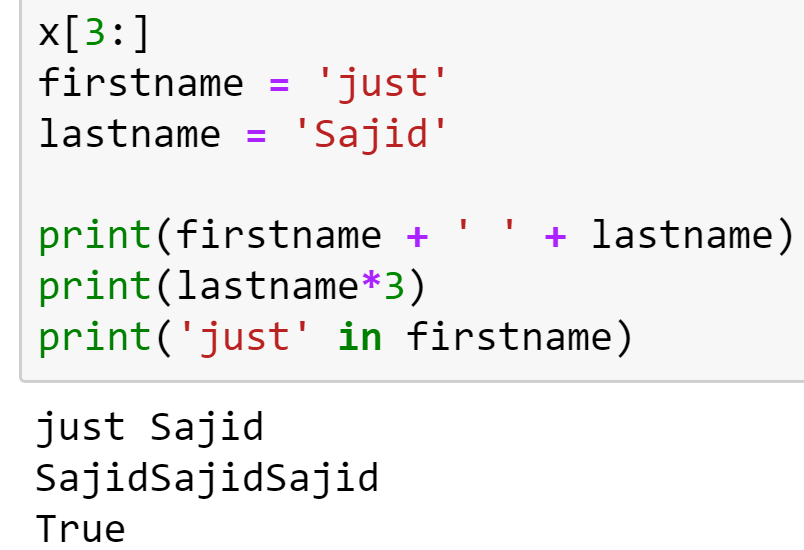
- Split returns a list of all the words in a string, or a list split on a specific character.

- * Make sure we convert objects to strings before concatenating.
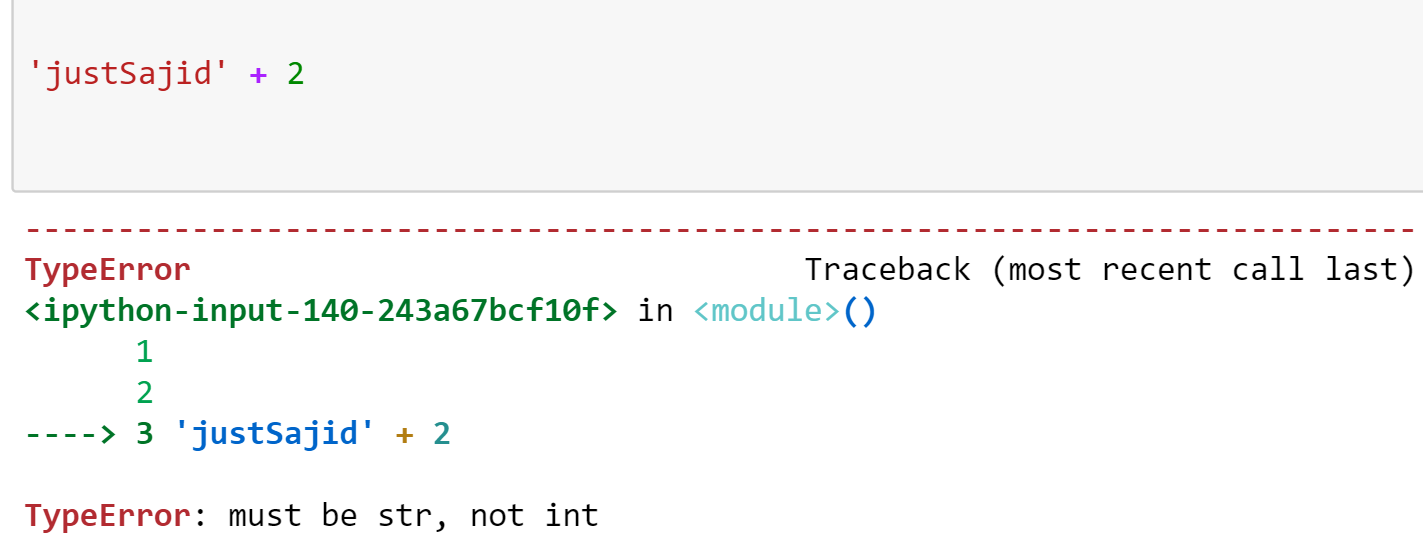
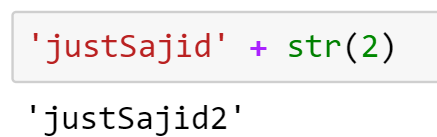
- Dictionaries associate keys with values.

- Iterate over all of the keys
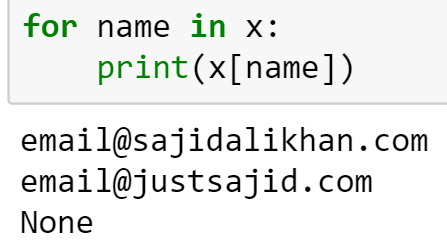
- Iterate over all of the values
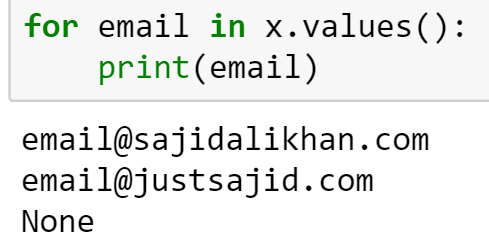
- We can unpack a sequence into different variables:
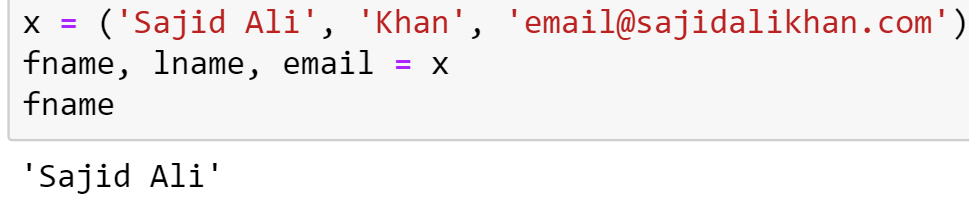
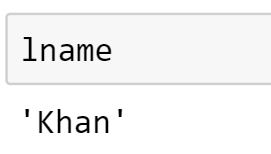
- Make sure the number of values you are unpacking matches the number of variables being assigned.
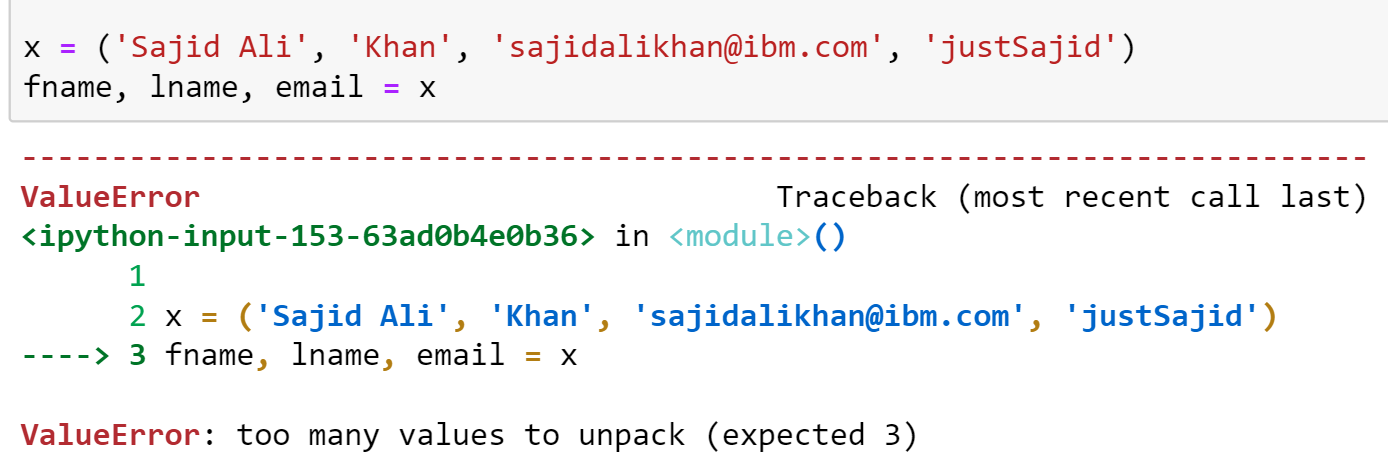
More on Strings
- Again, we have to use string type here;
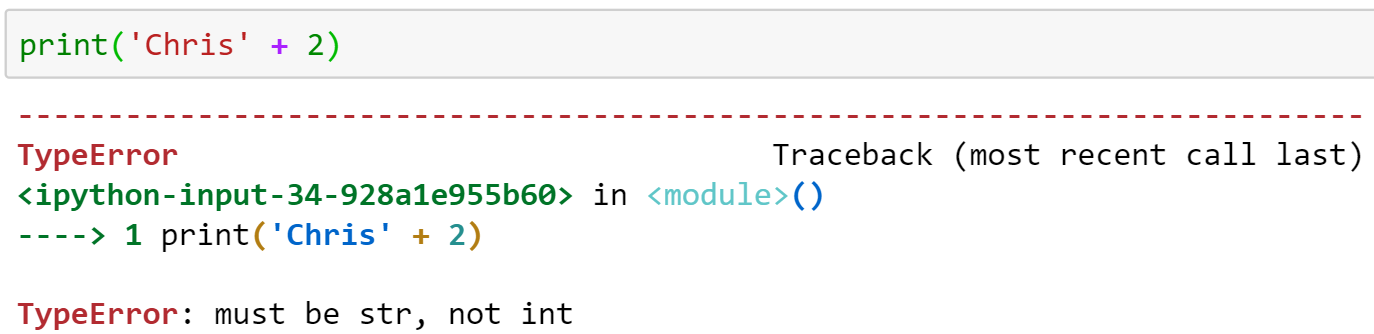
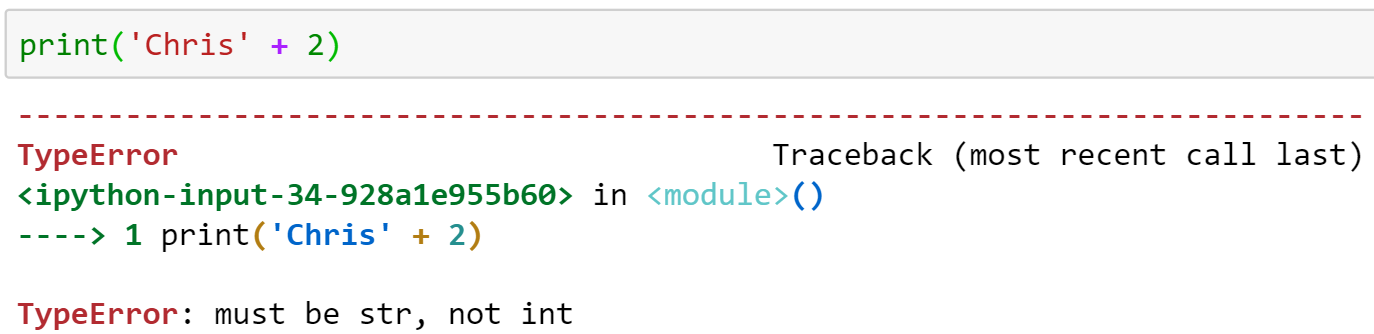
- Python has a built-in method for convenient string formatting.
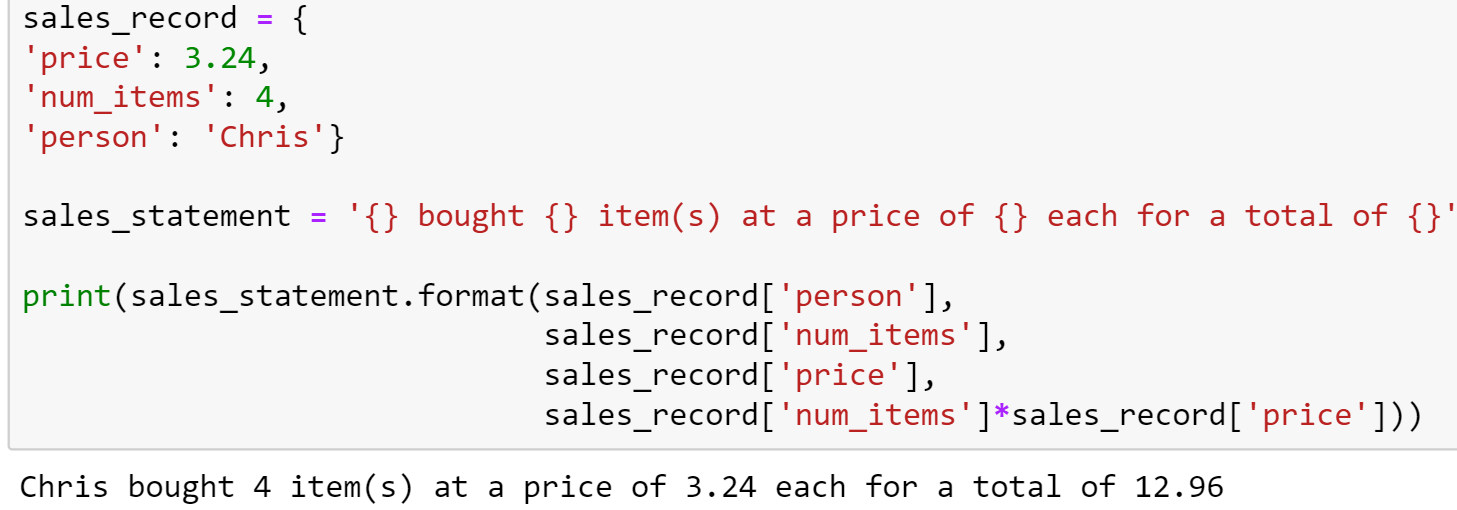
Dates and Times
For Dates and Times, we’ve to import “datetime” and “time” libraries;
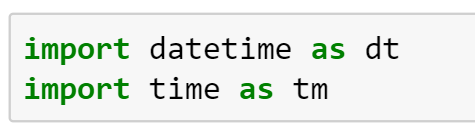
- “time” returns the current time in seconds since the Epoch. (January 1st, 1970)
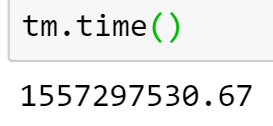
- Convert the timestamp to datetime.

- Handy datetime attributes.

- timedelta is a duration expressing the difference between two dates.

- date.today returns the current local date.
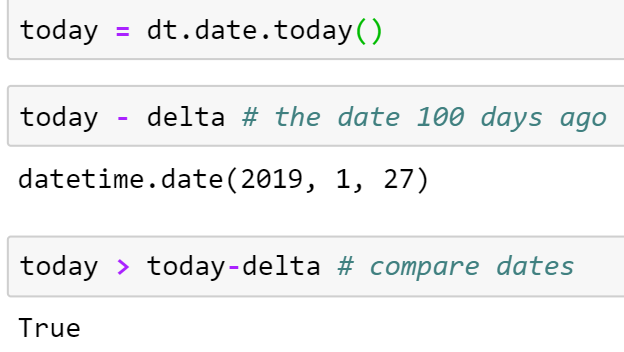
Objects and map()
- An example of a class in python
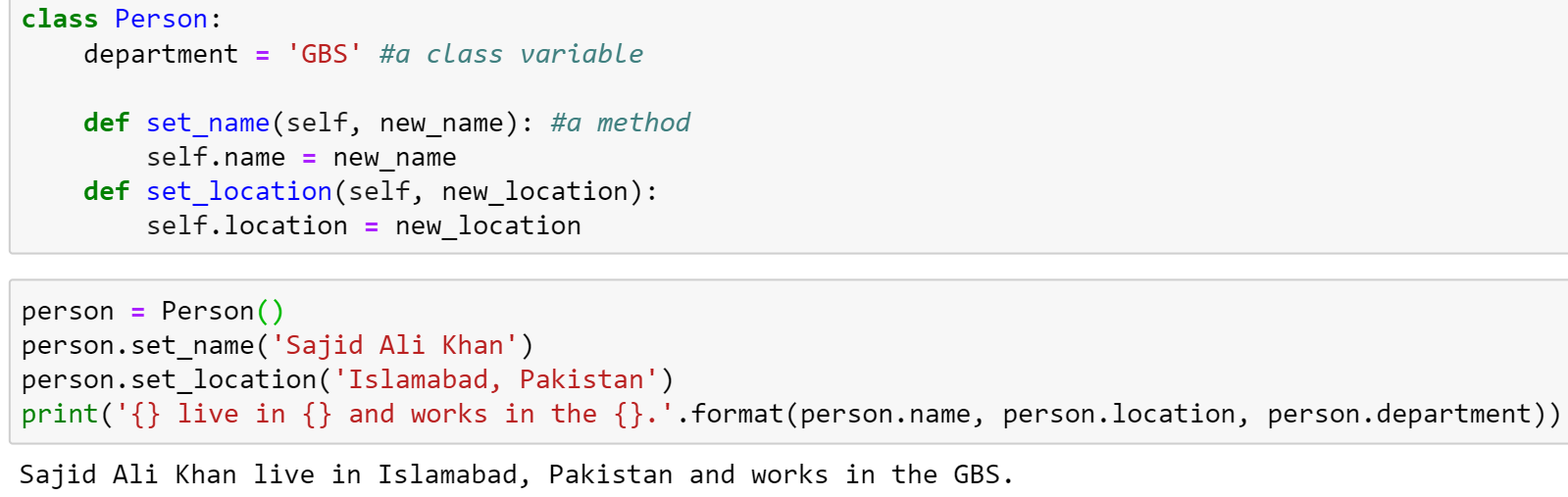
- An example of mapping the min function between two lists.
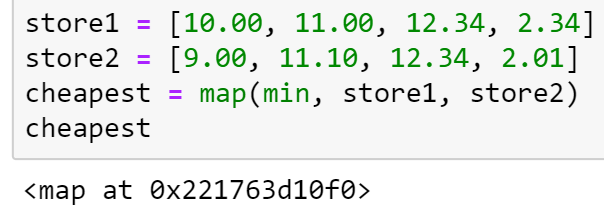
- Now let’s iterate through the map object to see the values.
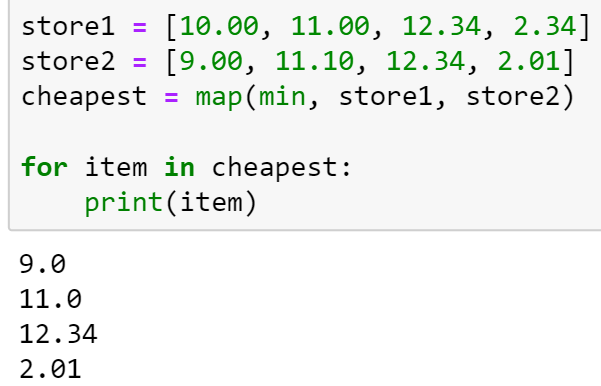
Lambda and List Comprehensions
Lambda’s are Python’s way of creating anonymous functions. These are the same as other functions, but they have no name. The intent is that they’re simple or short-lived and it’s easier just to write out the function in one line instead of going to the trouble of creating a named function. The return of a lambda is a function reference.
Note that we can’t have default values for lambda parameters and we can’t have complex logic inside of the lambda itself because we’re limited to a single expression.
So lambdas are really much more limited than full function definitions. But they’re very useful for simple little data cleaning tasks. And we can see lots of examples regarding that. So we should be able to read and write lambdas.
Here’s an example of lambda that takes in three parameters and adds the first two.
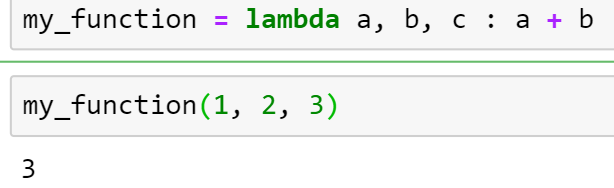
- Let’s iterate from 0 to 999 and return the even numbers.
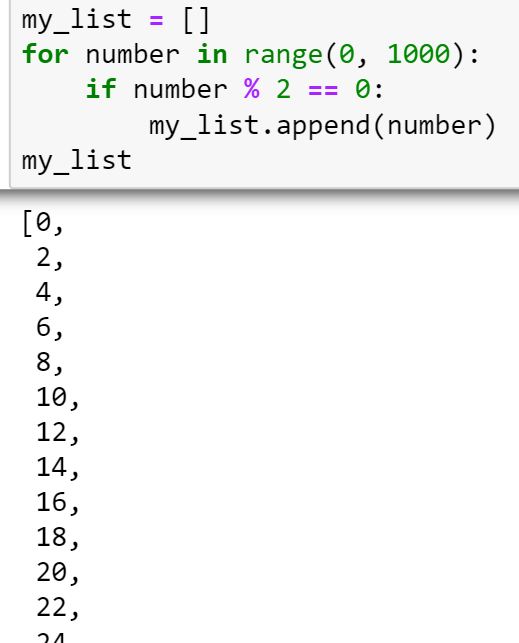
Numerical Python (NumPy)
- Let’s start with importing; “import numpy as np“
Creating Arrays
- Create a list and convert it to a numpy array
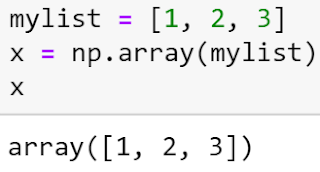
- We can also just pass in a list directly
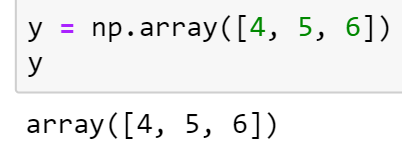
- Pass in a list of lists to create a multidimensional array.
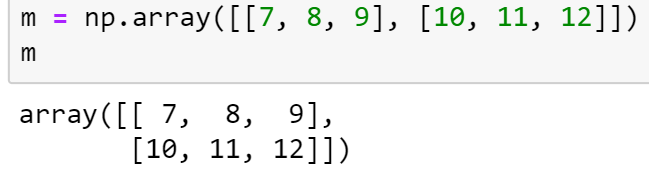
- Use the shape method to find the dimensions of the array. (rows, columns)
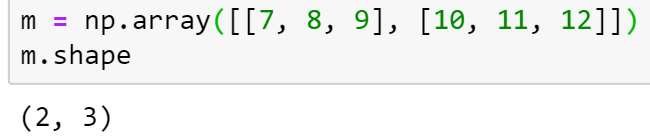
- Arrange returns evenly spaced values within a given interval.

- Reshape returns an array with the same data with a new shape.
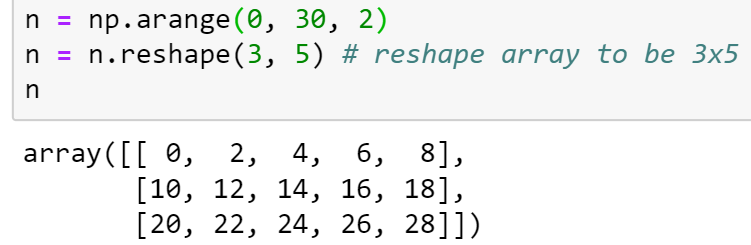
- Linspace returns evenly spaced numbers over a specified interval.

- Resize changes the shape and size of the array in-place.
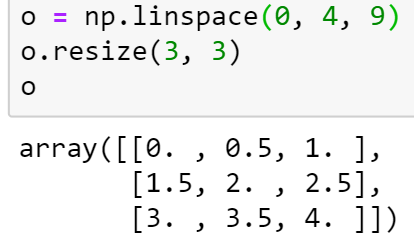
- Ones return a new array of given shape and type, filled with ones.
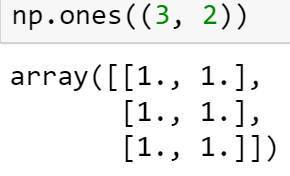
- zeros return a new array of given shape and type, filled with zeros.
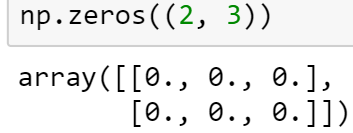
- Eye returns a 2-D array with ones on the diagonal and zeros elsewhere.
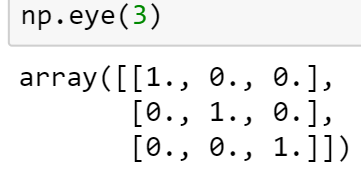
- Diag extracts a diagonal or constructs a diagonal array.
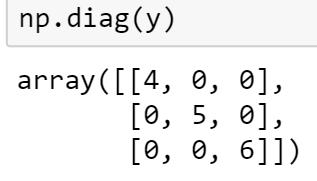
- Create an array using repeating list (or see np.tile)

- Repeat elements of an array using repeat.

Combining Arrays
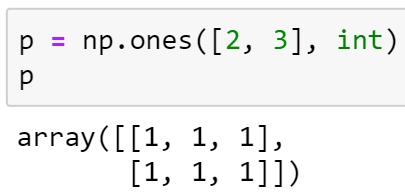
- Use vstack to stack arrays in sequence vertically (row wise).
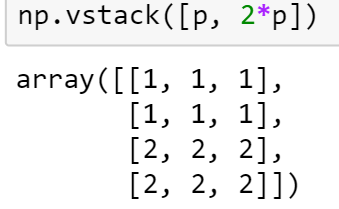
- Use hstack to stack arrays in sequence horizontally (column wise).
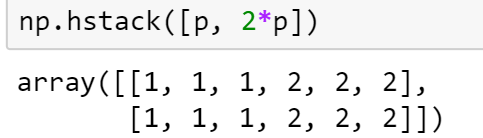
Operations
- Use +, -, *, / and ** to perform element-wise addition, subtraction, multiplication, division and power.


- elementwise power

- Dot Product

- number of rows of the array
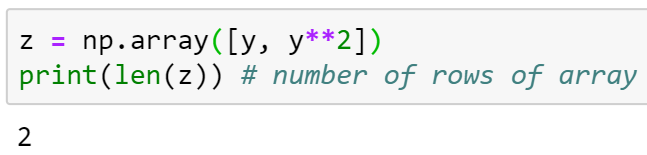
- Let’s look at transposing arrays. Transposing permutes the dimensions of the array.
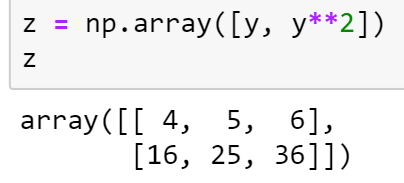
- The shape of the array z is (2,3) before transposing.
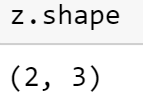
- Use .T to get the transpose.
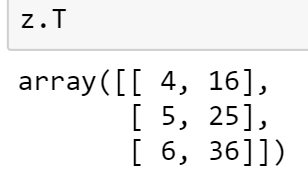
- The number of rows has swapped with the number of columns.
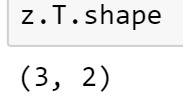
- Use .dtype to see the data type of the elements in the array.
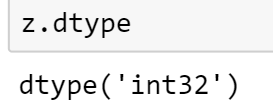
- Use .astype to cast to a specific type.
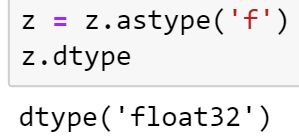
Math Functions
- Numpy has many built-in math functions that can be performed on arrays.
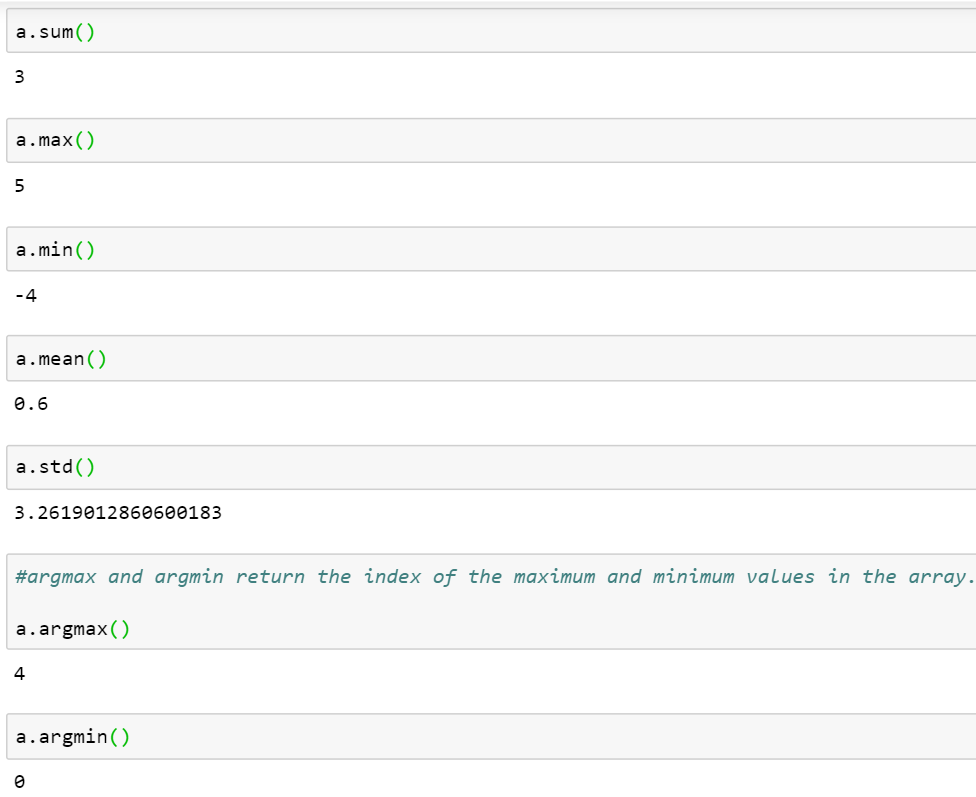
Indexing / Slicing

- Use bracket notation to get the value at a specific index. Remember that indexing starts at 0.
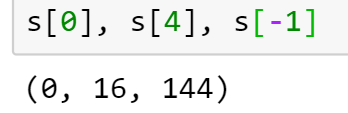
- Use “:” to indicate a range. array[start:stop]. (Leaving start or stop empty will default to the beginning/end of the array.)

- Use negatives to count from the back.

- A second “:” can be used to indicate step-size. array[start:stop:stepsize]. (Here we are starting 5th element from the end, and counting backwards by 2 until the beginning of the array is reached.)

- Let’s look at a multidimensional array.
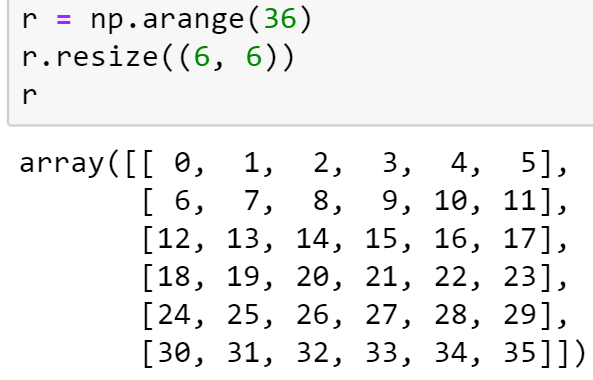
- Use bracket notation to slice: array[row, column]
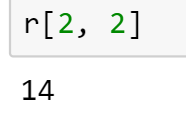
- And use “:” to select a range of rows or columns
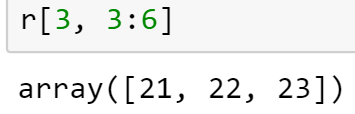
- Here we are selecting all the rows up to (and not including) row 2, and all the columns up to (and not including) the last column.
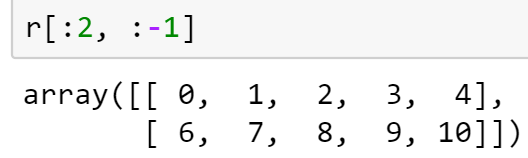
- This is a slice of the last row and only every other element.
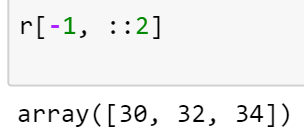
- We can also perform conditional indexing. Here we are selecting values from the array that are greater than 30. (Also see np.where)
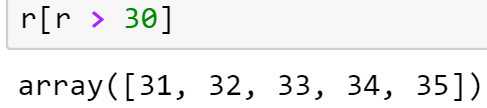
- Here we are assigning all values in the array that are greater than 30 to the value of 30.
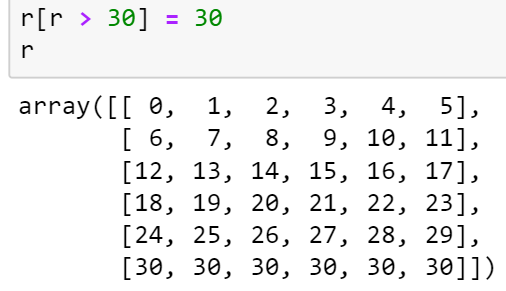
Copying Data
* Be careful with copying and modifying arrays in NumPy!
- r2 is a slice of r
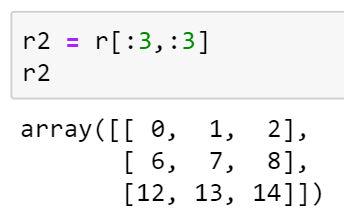
- Set this slice’s values to zero ([:] selects the entire array)
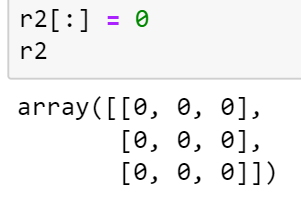
- r has also been changed!
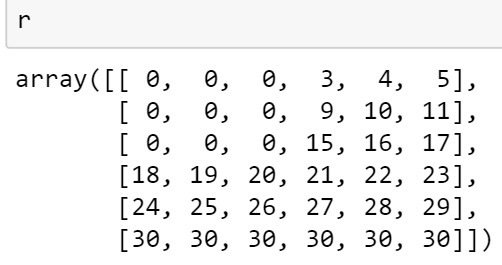
- To avoid this, use r.copy to create a copy that will not affect the original array
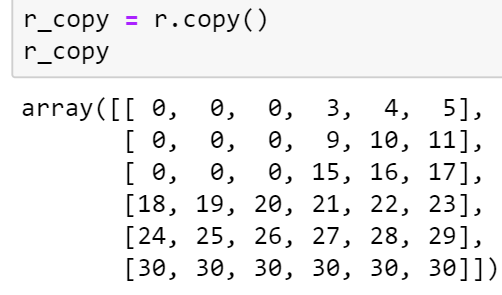
- Now when r_copy is modified, r will not be changed.
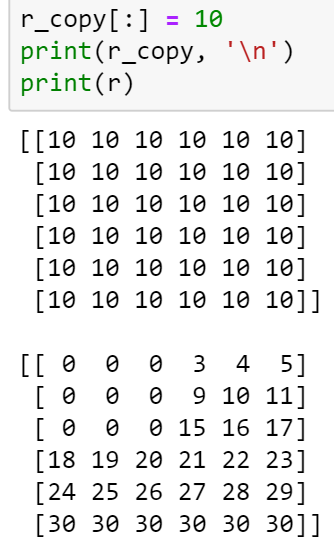
Iterating Over Arrays
- Let’s create a new 4 by 3 array of random numbers 0-9.
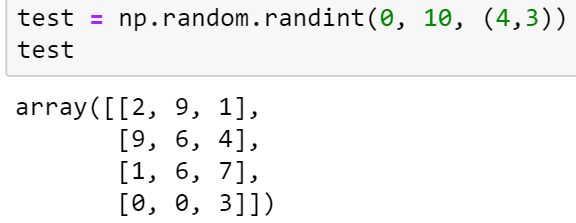
- Iterate by row
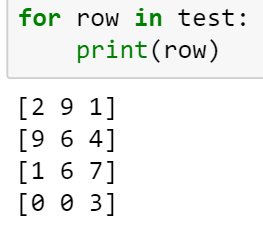
- Iterate by index:
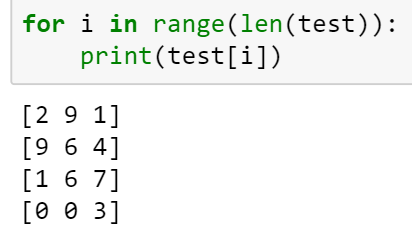
- Iterate by row and index:
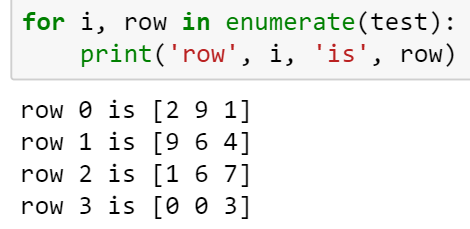
- Use zip to iterate over multiple iterables.
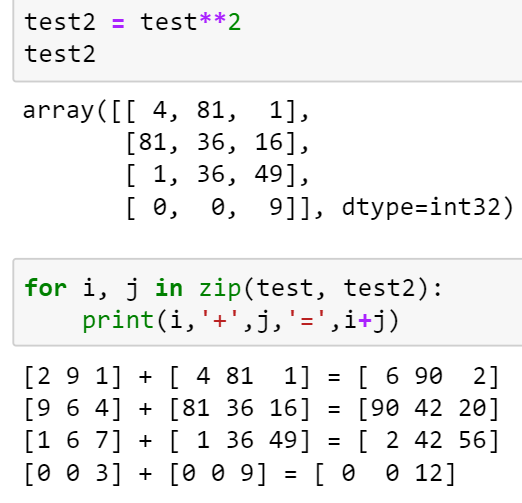
Conclusion
Further, one can perform further hands-on of different techniques like Reading and Writing data to/from csv/spreadsheets and also by using different python libraries. : – )